Migrated asdf library and all games / tests to gitea and added package.json to all projects.
31
asdf/Container.js
Normal file
@ -0,0 +1,31 @@
|
||||
class Container {
|
||||
constructor() {
|
||||
this.pos = { x: 0, y: 0};
|
||||
this.children = [];
|
||||
}
|
||||
|
||||
// Contrainer methods
|
||||
add (child) {
|
||||
this.children.push(child);
|
||||
return child;
|
||||
}
|
||||
|
||||
remove (child) {
|
||||
this.children = this.children.filter(c => c !== child);
|
||||
return child;
|
||||
}
|
||||
|
||||
map (f) {
|
||||
return this.children.map(f);
|
||||
}
|
||||
|
||||
update(dt, t) {
|
||||
this.children = this.children.filter(child => {
|
||||
if (child.update) {
|
||||
child.update(dt, t, this);
|
||||
}
|
||||
return child.dead ? false : true;
|
||||
});
|
||||
}
|
||||
}
|
||||
export default Container;
|
39
asdf/Game.js
Normal file
@ -0,0 +1,39 @@
|
||||
import Container from "./Container.js";
|
||||
import CanvasRenderer from "./renderer/CanvasRenderer.js";
|
||||
|
||||
const STEP = 1 / 60;
|
||||
const FRAME_MAX = 5 * STEP;
|
||||
|
||||
class Game {
|
||||
constructor (w, h, pixelated, parent = "#board") {
|
||||
this.w = w;
|
||||
this.h = h;
|
||||
this.renderer = new CanvasRenderer(w, h);
|
||||
document.querySelector(parent).appendChild(this.renderer.view);
|
||||
|
||||
if (pixelated) {
|
||||
this.renderer.setPixelated();
|
||||
}
|
||||
|
||||
this.scene = new Container();
|
||||
}
|
||||
|
||||
run(gameUpdate = () => {}) {
|
||||
let dt = 0;
|
||||
let last = 0;
|
||||
const loop = ms => {
|
||||
requestAnimationFrame(loop);
|
||||
|
||||
const t = ms / 1000;
|
||||
dt = Math.min(t - last, FRAME_MAX);
|
||||
last = t;
|
||||
|
||||
this.scene.update(dt, t);
|
||||
gameUpdate(dt, t);
|
||||
this.renderer.render(this.scene);
|
||||
};
|
||||
requestAnimationFrame(loop);
|
||||
}
|
||||
}
|
||||
|
||||
export default Game;
|
10
asdf/Sprite.js
Normal file
@ -0,0 +1,10 @@
|
||||
class Sprite {
|
||||
constructor(texture) {
|
||||
this.texture = texture;
|
||||
this.pos = { x: 0, y: 0 };
|
||||
this.anchor = { x: 0, y: 0 };
|
||||
this.scale = { x: 1, y: 1 };
|
||||
this.rotation = 0;
|
||||
}
|
||||
}
|
||||
export default Sprite;
|
8
asdf/Text.js
Normal file
@ -0,0 +1,8 @@
|
||||
class Text {
|
||||
constructor(text = "", style = {}) {
|
||||
this.pos = { x: 0, y: 0};
|
||||
this.text = text;
|
||||
this.style = style;
|
||||
}
|
||||
}
|
||||
export default Text;
|
7
asdf/Texture.js
Normal file
@ -0,0 +1,7 @@
|
||||
class Texture {
|
||||
constructor(url) {
|
||||
this.img = new Image();
|
||||
this.img.src = url;
|
||||
}
|
||||
}
|
||||
export default Texture;
|
12
asdf/TileSprite.js
Normal file
@ -0,0 +1,12 @@
|
||||
import Sprite from "./Sprite.js";
|
||||
|
||||
class TileSprite extends Sprite {
|
||||
constructor (texture, w, h) {
|
||||
super(texture);
|
||||
this.tileW = w;
|
||||
this.tileH = h;
|
||||
this.frame = { x: 0, y: 0 };
|
||||
}
|
||||
}
|
||||
|
||||
export default TileSprite;
|
59
asdf/controls/KeyControls.js
Normal file
@ -0,0 +1,59 @@
|
||||
class KeyControls {
|
||||
constructor() {
|
||||
this.keys = {};
|
||||
// Bind event handlers
|
||||
document.addEventListener("keydown", e => {
|
||||
if ([37,38,39,40].indexOf(e.which) >= 0) {
|
||||
e.preventDefault();
|
||||
}
|
||||
this.keys[e.which] = true;
|
||||
}, false);
|
||||
document.addEventListener('keyup', e => {
|
||||
this.keys[e.which] = false;
|
||||
}, false);
|
||||
}
|
||||
// Handle key actions
|
||||
get action() {
|
||||
// Spacebar
|
||||
return this.keys[32];
|
||||
}
|
||||
|
||||
get x () {
|
||||
// Arrow Left or A (WASD)
|
||||
if (this.keys[37] || this.keys[65]) {
|
||||
return -1;
|
||||
}
|
||||
// Arrow Right or D (WASD)
|
||||
if (this.keys[39] || this.keys[68]) {
|
||||
return 1;
|
||||
}
|
||||
return 0;
|
||||
}
|
||||
|
||||
get y () {
|
||||
// Arrow Up or W (WASD)
|
||||
if (this.keys[38] || this.keys[87]) {
|
||||
return -1;
|
||||
}
|
||||
// Arrow Down or S (WASD)
|
||||
if (this.keys[40] || this.keys[83]) {
|
||||
return 1;
|
||||
}
|
||||
return 0;
|
||||
}
|
||||
|
||||
key(key, value) {
|
||||
if (value !== undefined) {
|
||||
this.keys[key] = value;
|
||||
}
|
||||
return this.keys[key];
|
||||
}
|
||||
|
||||
reset() {
|
||||
for (let key in this.keys) {
|
||||
this.keys[key] = false;
|
||||
}
|
||||
}
|
||||
|
||||
}
|
||||
export default KeyControls;
|
46
asdf/controls/MouseControls.js
Normal file
@ -0,0 +1,46 @@
|
||||
class MouseControls {
|
||||
constructor(container) {
|
||||
this.el = container || document.body
|
||||
// State
|
||||
this.pos = {x: 0, y: 0};
|
||||
this.isDown = false;
|
||||
this.pressed = false;
|
||||
this.released = false;
|
||||
// Handlers
|
||||
document.addEventListener('mousemove', this.move.bind(this), false);
|
||||
document.addEventListener('mousedown', this.down.bind(this), false);
|
||||
document.addEventListener('mouseup', this.up.bind(this), false);
|
||||
}
|
||||
|
||||
mousePosFromEvent({ clientX, clientY }) {
|
||||
const { el, pos } = this;
|
||||
const rect = el.getBoundingClientRect();
|
||||
const xr = el.width / el.clientWidth;
|
||||
const yr = el.height / el.clientHeight;
|
||||
pos.x = (clientX - rect.left) * xr;
|
||||
pos.y = (clientY - rect.top) * yr;
|
||||
}
|
||||
|
||||
move(e) {
|
||||
this.mousePosFromEvent(e);
|
||||
}
|
||||
|
||||
down(e) {
|
||||
this.isDown = true;
|
||||
this.pressed = true;
|
||||
this.mousePosFromEvent(e);
|
||||
}
|
||||
|
||||
up() {
|
||||
this.isDown = false;
|
||||
this.released = true;
|
||||
}
|
||||
|
||||
update() {
|
||||
this.released = false;
|
||||
this.pressed = false;
|
||||
}
|
||||
|
||||
|
||||
}
|
||||
export default MouseControls;
|
13
asdf/index.html
Normal file
@ -0,0 +1,13 @@
|
||||
<!DOCTYPE html>
|
||||
<html lang="en">
|
||||
<head>
|
||||
<meta charset="UTF-8">
|
||||
<meta name="viewport" content="width=device-width, initial-scale=1.0">
|
||||
<meta http-equiv="X-UA-Compatible" content="ie=edge">
|
||||
<title>ASDF Framework</title>
|
||||
</head>
|
||||
<body>
|
||||
<h2>ASDF JS Framework</h2>
|
||||
<p>Nothing to browse here, just some shared files to make these games work.</p>
|
||||
</body>
|
||||
</html>
|
26
asdf/index.js
Normal file
@ -0,0 +1,26 @@
|
||||
import Container from "./Container.js";
|
||||
import CanvasRenderer from "./renderer/CanvasRenderer.js";
|
||||
import Game from "./Game.js";
|
||||
|
||||
import math from "./utilities/math.js";
|
||||
|
||||
import KeyControls from "./controls/KeyControls.js";
|
||||
import MouseControls from "./controls/MouseControls.js";
|
||||
|
||||
import Sprite from "./Sprite.js";
|
||||
import TileSprite from "./TileSprite.js";
|
||||
import Text from "./Text.js";
|
||||
import Texture from "./Texture.js";
|
||||
|
||||
export default {
|
||||
CanvasRenderer,
|
||||
Container,
|
||||
Game,
|
||||
math,
|
||||
KeyControls,
|
||||
MouseControls,
|
||||
Sprite,
|
||||
TileSprite,
|
||||
Text,
|
||||
Texture
|
||||
};
|
88
asdf/renderer/CanvasRenderer.js
Normal file
@ -0,0 +1,88 @@
|
||||
class CanvasRenderer {
|
||||
constructor(w, h) {
|
||||
const canvas = document.createElement("canvas");
|
||||
this.w = canvas.width = w;
|
||||
this.h = canvas.height = h;
|
||||
this.view = canvas;
|
||||
this.ctx = canvas.getContext("2d");
|
||||
this.ctx.textBaseLine = "top";
|
||||
}
|
||||
|
||||
setPixelated(){
|
||||
this.ctx['imageSmoothingEnabled'] = false; /* standard */
|
||||
this.ctx['mozImageSmoothingEnabled'] = false; /* Firefox */
|
||||
this.ctx['oImageSmoothingEnabled'] = false; /* Opera */
|
||||
this.ctx['webkitImageSmoothingEnabled'] = false; /* Safari */
|
||||
this.ctx['msImageSmoothingEnabled'] = false; /* IE */
|
||||
}
|
||||
|
||||
render(container, clear = true) {
|
||||
const { ctx } = this;
|
||||
function renderRec(container) {
|
||||
// Render container children
|
||||
container.children.forEach(child => {
|
||||
if (child.visible == false) {
|
||||
return;
|
||||
}
|
||||
|
||||
ctx.save();
|
||||
|
||||
// Draw the leaf node
|
||||
if (child.pos) {
|
||||
ctx.translate(Math.round(child.pos.x), Math.round(child.pos.y));
|
||||
}
|
||||
|
||||
if (child.anchor) {
|
||||
ctx.translate(child.anchor.x, child.anchor.y);
|
||||
}
|
||||
|
||||
if (child.scale) {
|
||||
ctx.scale(child.scale.x, child.scale.y);
|
||||
}
|
||||
|
||||
if (child.rotation) {
|
||||
const px = child.pivot ? child.pivot.x : 0;
|
||||
const py = child.pivot ? child.pivot.y : 0;
|
||||
ctx.translate(px, py);
|
||||
ctx.rotate(child.rotation);
|
||||
ctx.translate(-px, -py);
|
||||
}
|
||||
|
||||
if (child.text) {
|
||||
const { font, fill, align } = child.style;
|
||||
if (font) ctx.font = font;
|
||||
if (fill) ctx.fillStyle = fill;
|
||||
if (align) ctx.textAlign = align;
|
||||
ctx.fillText(child.text, 0,0);
|
||||
}
|
||||
|
||||
else if (child.texture) {
|
||||
const img = child.texture.img;
|
||||
if (child.tileW && child.tileH) {
|
||||
ctx.drawImage(
|
||||
img,
|
||||
child.frame.x * child.tileW,
|
||||
child.frame.y * child.tileH,
|
||||
child.tileW, child.tileH,
|
||||
0,0,
|
||||
child.tileW, child.tileH
|
||||
);
|
||||
} else {
|
||||
ctx.drawImage(img, 0, 0);
|
||||
}
|
||||
}
|
||||
|
||||
// Handle the child types
|
||||
if (child.children) {
|
||||
renderRec(child);
|
||||
}
|
||||
ctx.restore();
|
||||
})
|
||||
}
|
||||
if (clear) {
|
||||
ctx.clearRect(0,0,this.w,this.h);
|
||||
}
|
||||
renderRec(container);
|
||||
}
|
||||
}
|
||||
export default CanvasRenderer;
|
26
asdf/utilities/math.js
Normal file
@ -0,0 +1,26 @@
|
||||
function rand(min, max) {
|
||||
return Math.floor(randf(min, max));
|
||||
}
|
||||
|
||||
function randf(min, max) {
|
||||
if (max == null) {
|
||||
max = min || 1;
|
||||
min = 0;
|
||||
}
|
||||
return Math.random() * (max - min) + min;
|
||||
}
|
||||
|
||||
function randOneFrom(items) {
|
||||
return items[rand(items.length)];
|
||||
}
|
||||
|
||||
function randOneIn(max = 2) {
|
||||
return rand(0, max) === 0;
|
||||
}
|
||||
|
||||
export default {
|
||||
rand,
|
||||
randf,
|
||||
randOneFrom,
|
||||
randOneIn
|
||||
};
|
BIN
ctx-test/favicon.png
Normal file
After Width: | Height: | Size: 264 B |
20
ctx-test/index.html
Normal file
@ -0,0 +1,20 @@
|
||||
<html>
|
||||
<head>
|
||||
<meta charset="utf-8" />
|
||||
<link rel="icon" type="image/png" sizes="64x64" href="/data/favicon/favicon64.png">
|
||||
<link rel="icon" type="image/png" sizes="32x32" href="/data/favicon/favicon32.png">
|
||||
<link rel="icon" type="image/png" sizes="16x16" href="/data/favicon/favicon16.png">
|
||||
<link rel="apple-touch-icon" sizes="180x180" href="/data/favicon/apple-touch-icon.png">
|
||||
<link rel="manifest" href="/data/favicon/site.webmanifest">
|
||||
<link rel="mask-icon" href="/data/favicon/safari-pinned-tab.svg" color="#111111">
|
||||
<link rel="shortcut icon" href="/data/favicon/favicon.ico">
|
||||
<title>Game</title>
|
||||
<link rel="stylesheet" href="res/main.css" />
|
||||
</head>
|
||||
<body>
|
||||
<div id="board">
|
||||
<canvas width="640" height="480"></canvas>
|
||||
</div>
|
||||
<script type="module" src="src/main.js"></script>
|
||||
</body>
|
||||
</html>
|
59
ctx-test/lib/KeyControls.js
Normal file
@ -0,0 +1,59 @@
|
||||
class KeyControls {
|
||||
constructor() {
|
||||
this.keys = {};
|
||||
// Bind event handlers
|
||||
document.addEventListener("keydown", e => {
|
||||
if ([37,38,39,40].indexOf(e.which) >= 0) {
|
||||
e.preventDefault();
|
||||
}
|
||||
this.keys[e.which] = true;
|
||||
}, false);
|
||||
document.addEventListener('keyup', e => {
|
||||
this.keys[e.which] = false;
|
||||
}, false);
|
||||
}
|
||||
// Handle key actions
|
||||
get action() {
|
||||
// Spacebar
|
||||
return this.keys[32];
|
||||
}
|
||||
|
||||
get x () {
|
||||
// Arrow Left or A (WASD)
|
||||
if (this.keys[37] || this.keys[65]) {
|
||||
return -1;
|
||||
}
|
||||
// Arrow Right or D (WASD)
|
||||
if (this.keys[39] || this.keys[68]) {
|
||||
return 1;
|
||||
}
|
||||
return 0;
|
||||
}
|
||||
|
||||
get y () {
|
||||
// Arrow Up or W (WASD)
|
||||
if (this.keys[38] || this.keys[87]) {
|
||||
return -1;
|
||||
}
|
||||
// Arrow Down or S (WASD)
|
||||
if (this.keys[40] || this.keys[83]) {
|
||||
return 1;
|
||||
}
|
||||
return 0;
|
||||
}
|
||||
|
||||
key(key, value) {
|
||||
if (value !== undefined) {
|
||||
this.keys[key] = value;
|
||||
}
|
||||
return this.keys[key];
|
||||
}
|
||||
|
||||
reset() {
|
||||
for (let key in this.keys) {
|
||||
this.keys[key] = false;
|
||||
}
|
||||
}
|
||||
|
||||
}
|
||||
export default KeyControls;
|
46
ctx-test/lib/MouseControls.js
Normal file
@ -0,0 +1,46 @@
|
||||
class MouseControls {
|
||||
constructor(container) {
|
||||
this.el = container || document.body
|
||||
// State
|
||||
this.pos = {x: 0, y: 0};
|
||||
this.isDown = false;
|
||||
this.pressed = false;
|
||||
this.released = false;
|
||||
// Handlers
|
||||
document.addEventListener('mousemove', this.move.bind(this), false);
|
||||
document.addEventListener('mousedown', this.down.bind(this), false);
|
||||
document.addEventListener('mouseup', this.up.bind(this), false);
|
||||
}
|
||||
|
||||
mousePosFromEvent({ clientX, clientY }) {
|
||||
const { el, pos } = this;
|
||||
const rect = el.getBoundingClientRect();
|
||||
const xr = el.width / el.clientWidth;
|
||||
const yr = el.height / el.clientHeight;
|
||||
pos.x = (clientX - rect.left) * xr;
|
||||
pos.y = (clientY - rect.top) * yr;
|
||||
}
|
||||
|
||||
move(e) {
|
||||
this.mousePosFromEvent(e);
|
||||
}
|
||||
|
||||
down(e) {
|
||||
this.isDown = true;
|
||||
this.pressed = true;
|
||||
this.mousePosFromEvent(e);
|
||||
}
|
||||
|
||||
up() {
|
||||
this.isDown = false;
|
||||
this.released = true;
|
||||
}
|
||||
|
||||
update() {
|
||||
this.released = false;
|
||||
this.pressed = false;
|
||||
}
|
||||
|
||||
|
||||
}
|
||||
export default MouseControls;
|
20
ctx-test/package.json
Normal file
@ -0,0 +1,20 @@
|
||||
{
|
||||
"name": "asdf-ctx-test",
|
||||
"version": "1.0.0",
|
||||
"description": "Canvas renderer test",
|
||||
"main": "index.html",
|
||||
"directories": {
|
||||
"lib": "lib"
|
||||
},
|
||||
"scripts": {
|
||||
"test": "na"
|
||||
},
|
||||
"keywords": [
|
||||
"canvas",
|
||||
"renderer",
|
||||
"test",
|
||||
"asdf"
|
||||
],
|
||||
"author": "Arne van Iterson",
|
||||
"license": "ISC"
|
||||
}
|
BIN
ctx-test/res/images/rc2000.png
Normal file
After Width: | Height: | Size: 57 KiB |
BIN
ctx-test/res/images/rick.png
Normal file
After Width: | Height: | Size: 44 KiB |
17
ctx-test/res/main.css
Normal file
@ -0,0 +1,17 @@
|
||||
body {
|
||||
background-color: rgba(42, 42, 46, 1);
|
||||
text-align: center;
|
||||
|
||||
-moz-user-select: none;
|
||||
-webkit-user-select: none;
|
||||
-ms-user-select: none;
|
||||
user-select: none;
|
||||
}
|
||||
#board {
|
||||
position: relative;
|
||||
background-color: #111;
|
||||
width: 640px;
|
||||
height: 480px;
|
||||
margin: auto;
|
||||
border: 5px solid whitesmoke;
|
||||
}
|
34
ctx-test/src/main.js
Normal file
@ -0,0 +1,34 @@
|
||||
import asdf from "../../asdf/index.js";
|
||||
const { Container, CanvasRenderer, KeyControls, MouseControls, Text, Texture, Sprite } = asdf;
|
||||
|
||||
const canvas = document.querySelector("#board canvas");
|
||||
const ctx = canvas.getContext("2d");
|
||||
const { width: w, height: h } = canvas;
|
||||
console.log(canvas);
|
||||
|
||||
// Setup Code
|
||||
const controls = new KeyControls();
|
||||
const mouse = new MouseControls(canvas);
|
||||
let x = w / 2;
|
||||
let y = h / 2;
|
||||
|
||||
// Looping Code
|
||||
function loopme(ms) {
|
||||
requestAnimationFrame(loopme);
|
||||
ctx.fillStyle = '#111'
|
||||
// Game logic code
|
||||
ctx.save();
|
||||
ctx.fillRect(0,0,w,h);
|
||||
x = mouse.pos.x * 1;
|
||||
y = mouse.pos.y * 1;
|
||||
if (mouse.isDown) {
|
||||
ctx.fillStyle = '#00f'
|
||||
} else {
|
||||
ctx.fillStyle = '#f00'
|
||||
}
|
||||
ctx.fillRect(x, y, 5, 5);
|
||||
ctx.fillRect(0, 0, 5, 5);
|
||||
ctx.restore();
|
||||
mouse.update();
|
||||
}
|
||||
requestAnimationFrame(loopme);
|
BIN
lib-test/favicon.png
Normal file
After Width: | Height: | Size: 264 B |
20
lib-test/index.html
Normal file
@ -0,0 +1,20 @@
|
||||
<html>
|
||||
<head>
|
||||
<meta charset="utf-8" />
|
||||
<link rel="icon" type="image/png" sizes="64x64" href="/data/favicon/favicon64.png">
|
||||
<link rel="icon" type="image/png" sizes="32x32" href="/data/favicon/favicon32.png">
|
||||
<link rel="icon" type="image/png" sizes="16x16" href="/data/favicon/favicon16.png">
|
||||
<link rel="apple-touch-icon" sizes="180x180" href="/data/favicon/apple-touch-icon.png">
|
||||
<link rel="manifest" href="/data/favicon/site.webmanifest">
|
||||
<link rel="mask-icon" href="/data/favicon/safari-pinned-tab.svg" color="#111111">
|
||||
<link rel="shortcut icon" href="/data/favicon/favicon.ico">
|
||||
<title>Game</title>
|
||||
<link rel="stylesheet" href="res/main.css" />
|
||||
</head>
|
||||
<body>
|
||||
<div id="board">
|
||||
<!-- Renderer will push content here -->
|
||||
</div>
|
||||
<script type="module" src="src/main.js"></script>
|
||||
</body>
|
||||
</html>
|
19
lib-test/package.json
Normal file
@ -0,0 +1,19 @@
|
||||
{
|
||||
"name": "asdf-lib-test",
|
||||
"version": "1.0.0",
|
||||
"description": "Library test",
|
||||
"main": "index.html",
|
||||
"directories": {
|
||||
"lib": "lib"
|
||||
},
|
||||
"scripts": {
|
||||
"test": "na"
|
||||
},
|
||||
"keywords": [
|
||||
"library",
|
||||
"test",
|
||||
"asdf"
|
||||
],
|
||||
"author": "Arne van Iterson",
|
||||
"license": "ISC"
|
||||
}
|
BIN
lib-test/res/images/building.png
Normal file
After Width: | Height: | Size: 1.2 KiB |
BIN
lib-test/res/images/characters.png
Normal file
After Width: | Height: | Size: 12 KiB |
BIN
lib-test/res/images/rc2000.png
Normal file
After Width: | Height: | Size: 57 KiB |
BIN
lib-test/res/images/rick.png
Normal file
After Width: | Height: | Size: 44 KiB |
BIN
lib-test/res/images/spaceship.png
Normal file
After Width: | Height: | Size: 405 B |
BIN
lib-test/res/images/tiles_packed.png
Normal file
After Width: | Height: | Size: 20 KiB |
17
lib-test/res/main.css
Normal file
@ -0,0 +1,17 @@
|
||||
body {
|
||||
background-color: rgba(42, 42, 46, 1);
|
||||
text-align: center;
|
||||
|
||||
-moz-user-select: none;
|
||||
-webkit-user-select: none;
|
||||
-ms-user-select: none;
|
||||
user-select: none;
|
||||
}
|
||||
#board {
|
||||
position: relative;
|
||||
background-color: #111;
|
||||
width: max-content;
|
||||
height: auto;
|
||||
margin: auto;
|
||||
border: 5px solid whitesmoke;
|
||||
}
|
48
lib-test/src/main.js
Normal file
@ -0,0 +1,48 @@
|
||||
import asdf from "../../asdf/index.js";
|
||||
const { Game, Container, CanvasRenderer, math, KeyControls, MouseControls, Text, Texture, Sprite } = asdf;
|
||||
|
||||
const game = new Game(640, 320, false);
|
||||
const { scene, w, h } = game;
|
||||
|
||||
const buildings = scene.add(new Container());
|
||||
const makeRandom = (b, x) => {
|
||||
b.scale.x = math.randf(1,3);
|
||||
b.scale.y = math.randf(1,3);
|
||||
b.pos.x = x;
|
||||
b.pos.y = h - b.scale.y * 64;
|
||||
};
|
||||
|
||||
for (let x = 0; x < 50; x++) {
|
||||
const b = buildings.add(new Sprite(new Texture("res/images/building.png")));
|
||||
makeRandom(b, math.rand(w));
|
||||
}
|
||||
|
||||
var rotation = 0;
|
||||
const ship = new Sprite(new Texture("res/images/spaceship.png"));
|
||||
ship.pivot = { x: 16, y: 16 };
|
||||
scene.add(ship);
|
||||
|
||||
|
||||
game.run((dt ,t) => {
|
||||
ship.update = function(dt, t) {
|
||||
const {scale} = this;
|
||||
scale.x = Math.abs(Math.sin(t)) + 1;
|
||||
scale.y = Math.abs(Math.sin(t)) + 1;
|
||||
|
||||
rotation = rotation + 0.2;
|
||||
ship.rotation = rotation;
|
||||
|
||||
ship.pos.y = (Math.abs(Math.sin(t))) * 50 + (h / 2) - 16;
|
||||
ship.pos.x += dt * 50;
|
||||
if (ship.pos.x > w) {
|
||||
ship.pos.x = -32;
|
||||
}
|
||||
}
|
||||
|
||||
buildings.map(b => {
|
||||
b.pos.x -= 100 * dt;
|
||||
if (b.pos.x < -80) {
|
||||
makeRandom(b,w);
|
||||
}
|
||||
});
|
||||
});
|
5
list.js
Normal file
@ -0,0 +1,5 @@
|
||||
var serveIndex = require('serve-index')
|
||||
|
||||
require('budo').cli(process.argv.slice(2), {
|
||||
middleware: serveIndex(__dirname)
|
||||
})
|
15
node_modules/.bin/atob
generated
vendored
Normal file
@ -0,0 +1,15 @@
|
||||
#!/bin/sh
|
||||
basedir=$(dirname "$(echo "$0" | sed -e 's,\\,/,g')")
|
||||
|
||||
case `uname` in
|
||||
*CYGWIN*) basedir=`cygpath -w "$basedir"`;;
|
||||
esac
|
||||
|
||||
if [ -x "$basedir/node" ]; then
|
||||
"$basedir/node" "$basedir/../atob/bin/atob.js" "$@"
|
||||
ret=$?
|
||||
else
|
||||
node "$basedir/../atob/bin/atob.js" "$@"
|
||||
ret=$?
|
||||
fi
|
||||
exit $ret
|
7
node_modules/.bin/atob.cmd
generated
vendored
Normal file
@ -0,0 +1,7 @@
|
||||
@IF EXIST "%~dp0\node.exe" (
|
||||
"%~dp0\node.exe" "%~dp0\..\atob\bin\atob.js" %*
|
||||
) ELSE (
|
||||
@SETLOCAL
|
||||
@SET PATHEXT=%PATHEXT:;.JS;=;%
|
||||
node "%~dp0\..\atob\bin\atob.js" %*
|
||||
)
|
15
node_modules/.bin/browserify
generated
vendored
Normal file
@ -0,0 +1,15 @@
|
||||
#!/bin/sh
|
||||
basedir=$(dirname "$(echo "$0" | sed -e 's,\\,/,g')")
|
||||
|
||||
case `uname` in
|
||||
*CYGWIN*) basedir=`cygpath -w "$basedir"`;;
|
||||
esac
|
||||
|
||||
if [ -x "$basedir/node" ]; then
|
||||
"$basedir/node" "$basedir/../browserify/bin/cmd.js" "$@"
|
||||
ret=$?
|
||||
else
|
||||
node "$basedir/../browserify/bin/cmd.js" "$@"
|
||||
ret=$?
|
||||
fi
|
||||
exit $ret
|
7
node_modules/.bin/browserify.cmd
generated
vendored
Normal file
@ -0,0 +1,7 @@
|
||||
@IF EXIST "%~dp0\node.exe" (
|
||||
"%~dp0\node.exe" "%~dp0\..\browserify\bin\cmd.js" %*
|
||||
) ELSE (
|
||||
@SETLOCAL
|
||||
@SET PATHEXT=%PATHEXT:;.JS;=;%
|
||||
node "%~dp0\..\browserify\bin\cmd.js" %*
|
||||
)
|
15
node_modules/.bin/budo
generated
vendored
Normal file
@ -0,0 +1,15 @@
|
||||
#!/bin/sh
|
||||
basedir=$(dirname "$(echo "$0" | sed -e 's,\\,/,g')")
|
||||
|
||||
case `uname` in
|
||||
*CYGWIN*) basedir=`cygpath -w "$basedir"`;;
|
||||
esac
|
||||
|
||||
if [ -x "$basedir/node" ]; then
|
||||
"$basedir/node" "$basedir/../budo/bin/cmd.js" "$@"
|
||||
ret=$?
|
||||
else
|
||||
node "$basedir/../budo/bin/cmd.js" "$@"
|
||||
ret=$?
|
||||
fi
|
||||
exit $ret
|
7
node_modules/.bin/budo.cmd
generated
vendored
Normal file
@ -0,0 +1,7 @@
|
||||
@IF EXIST "%~dp0\node.exe" (
|
||||
"%~dp0\node.exe" "%~dp0\..\budo\bin\cmd.js" %*
|
||||
) ELSE (
|
||||
@SETLOCAL
|
||||
@SET PATHEXT=%PATHEXT:;.JS;=;%
|
||||
node "%~dp0\..\budo\bin\cmd.js" %*
|
||||
)
|
15
node_modules/.bin/garnish
generated
vendored
Normal file
@ -0,0 +1,15 @@
|
||||
#!/bin/sh
|
||||
basedir=$(dirname "$(echo "$0" | sed -e 's,\\,/,g')")
|
||||
|
||||
case `uname` in
|
||||
*CYGWIN*) basedir=`cygpath -w "$basedir"`;;
|
||||
esac
|
||||
|
||||
if [ -x "$basedir/node" ]; then
|
||||
"$basedir/node" "$basedir/../garnish/bin/cmd.js" "$@"
|
||||
ret=$?
|
||||
else
|
||||
node "$basedir/../garnish/bin/cmd.js" "$@"
|
||||
ret=$?
|
||||
fi
|
||||
exit $ret
|
7
node_modules/.bin/garnish.cmd
generated
vendored
Normal file
@ -0,0 +1,7 @@
|
||||
@IF EXIST "%~dp0\node.exe" (
|
||||
"%~dp0\node.exe" "%~dp0\..\garnish\bin\cmd.js" %*
|
||||
) ELSE (
|
||||
@SETLOCAL
|
||||
@SET PATHEXT=%PATHEXT:;.JS;=;%
|
||||
node "%~dp0\..\garnish\bin\cmd.js" %*
|
||||
)
|
15
node_modules/.bin/has-ansi
generated
vendored
Normal file
@ -0,0 +1,15 @@
|
||||
#!/bin/sh
|
||||
basedir=$(dirname "$(echo "$0" | sed -e 's,\\,/,g')")
|
||||
|
||||
case `uname` in
|
||||
*CYGWIN*) basedir=`cygpath -w "$basedir"`;;
|
||||
esac
|
||||
|
||||
if [ -x "$basedir/node" ]; then
|
||||
"$basedir/node" "$basedir/../has-ansi/cli.js" "$@"
|
||||
ret=$?
|
||||
else
|
||||
node "$basedir/../has-ansi/cli.js" "$@"
|
||||
ret=$?
|
||||
fi
|
||||
exit $ret
|
7
node_modules/.bin/has-ansi.cmd
generated
vendored
Normal file
@ -0,0 +1,7 @@
|
||||
@IF EXIST "%~dp0\node.exe" (
|
||||
"%~dp0\node.exe" "%~dp0\..\has-ansi\cli.js" %*
|
||||
) ELSE (
|
||||
@SETLOCAL
|
||||
@SET PATHEXT=%PATHEXT:;.JS;=;%
|
||||
node "%~dp0\..\has-ansi\cli.js" %*
|
||||
)
|
15
node_modules/.bin/mime
generated
vendored
Normal file
@ -0,0 +1,15 @@
|
||||
#!/bin/sh
|
||||
basedir=$(dirname "$(echo "$0" | sed -e 's,\\,/,g')")
|
||||
|
||||
case `uname` in
|
||||
*CYGWIN*) basedir=`cygpath -w "$basedir"`;;
|
||||
esac
|
||||
|
||||
if [ -x "$basedir/node" ]; then
|
||||
"$basedir/node" "$basedir/../mime/cli.js" "$@"
|
||||
ret=$?
|
||||
else
|
||||
node "$basedir/../mime/cli.js" "$@"
|
||||
ret=$?
|
||||
fi
|
||||
exit $ret
|
7
node_modules/.bin/mime.cmd
generated
vendored
Normal file
@ -0,0 +1,7 @@
|
||||
@IF EXIST "%~dp0\node.exe" (
|
||||
"%~dp0\node.exe" "%~dp0\..\mime\cli.js" %*
|
||||
) ELSE (
|
||||
@SETLOCAL
|
||||
@SET PATHEXT=%PATHEXT:;.JS;=;%
|
||||
node "%~dp0\..\mime\cli.js" %*
|
||||
)
|
15
node_modules/.bin/semver
generated
vendored
Normal file
@ -0,0 +1,15 @@
|
||||
#!/bin/sh
|
||||
basedir=$(dirname "$(echo "$0" | sed -e 's,\\,/,g')")
|
||||
|
||||
case `uname` in
|
||||
*CYGWIN*) basedir=`cygpath -w "$basedir"`;;
|
||||
esac
|
||||
|
||||
if [ -x "$basedir/node" ]; then
|
||||
"$basedir/node" "$basedir/../semver/bin/semver" "$@"
|
||||
ret=$?
|
||||
else
|
||||
node "$basedir/../semver/bin/semver" "$@"
|
||||
ret=$?
|
||||
fi
|
||||
exit $ret
|
7
node_modules/.bin/semver.cmd
generated
vendored
Normal file
@ -0,0 +1,7 @@
|
||||
@IF EXIST "%~dp0\node.exe" (
|
||||
"%~dp0\node.exe" "%~dp0\..\semver\bin\semver" %*
|
||||
) ELSE (
|
||||
@SETLOCAL
|
||||
@SET PATHEXT=%PATHEXT:;.JS;=;%
|
||||
node "%~dp0\..\semver\bin\semver" %*
|
||||
)
|
15
node_modules/.bin/supports-color
generated
vendored
Normal file
@ -0,0 +1,15 @@
|
||||
#!/bin/sh
|
||||
basedir=$(dirname "$(echo "$0" | sed -e 's,\\,/,g')")
|
||||
|
||||
case `uname` in
|
||||
*CYGWIN*) basedir=`cygpath -w "$basedir"`;;
|
||||
esac
|
||||
|
||||
if [ -x "$basedir/node" ]; then
|
||||
"$basedir/node" "$basedir/../supports-color/cli.js" "$@"
|
||||
ret=$?
|
||||
else
|
||||
node "$basedir/../supports-color/cli.js" "$@"
|
||||
ret=$?
|
||||
fi
|
||||
exit $ret
|
7
node_modules/.bin/supports-color.cmd
generated
vendored
Normal file
@ -0,0 +1,7 @@
|
||||
@IF EXIST "%~dp0\node.exe" (
|
||||
"%~dp0\node.exe" "%~dp0\..\supports-color\cli.js" %*
|
||||
) ELSE (
|
||||
@SETLOCAL
|
||||
@SET PATHEXT=%PATHEXT:;.JS;=;%
|
||||
node "%~dp0\..\supports-color\cli.js" %*
|
||||
)
|
15
node_modules/.bin/watchify
generated
vendored
Normal file
@ -0,0 +1,15 @@
|
||||
#!/bin/sh
|
||||
basedir=$(dirname "$(echo "$0" | sed -e 's,\\,/,g')")
|
||||
|
||||
case `uname` in
|
||||
*CYGWIN*) basedir=`cygpath -w "$basedir"`;;
|
||||
esac
|
||||
|
||||
if [ -x "$basedir/node" ]; then
|
||||
"$basedir/node" "$basedir/../watchify/bin/cmd.js" "$@"
|
||||
ret=$?
|
||||
else
|
||||
node "$basedir/../watchify/bin/cmd.js" "$@"
|
||||
ret=$?
|
||||
fi
|
||||
exit $ret
|
7
node_modules/.bin/watchify.cmd
generated
vendored
Normal file
@ -0,0 +1,7 @@
|
||||
@IF EXIST "%~dp0\node.exe" (
|
||||
"%~dp0\node.exe" "%~dp0\..\watchify\bin\cmd.js" %*
|
||||
) ELSE (
|
||||
@SETLOCAL
|
||||
@SET PATHEXT=%PATHEXT:;.JS;=;%
|
||||
node "%~dp0\..\watchify\bin\cmd.js" %*
|
||||
)
|
15
node_modules/.bin/which
generated
vendored
Normal file
@ -0,0 +1,15 @@
|
||||
#!/bin/sh
|
||||
basedir=$(dirname "$(echo "$0" | sed -e 's,\\,/,g')")
|
||||
|
||||
case `uname` in
|
||||
*CYGWIN*) basedir=`cygpath -w "$basedir"`;;
|
||||
esac
|
||||
|
||||
if [ -x "$basedir/node" ]; then
|
||||
"$basedir/node" "$basedir/../which/bin/which" "$@"
|
||||
ret=$?
|
||||
else
|
||||
node "$basedir/../which/bin/which" "$@"
|
||||
ret=$?
|
||||
fi
|
||||
exit $ret
|
7
node_modules/.bin/which.cmd
generated
vendored
Normal file
@ -0,0 +1,7 @@
|
||||
@IF EXIST "%~dp0\node.exe" (
|
||||
"%~dp0\node.exe" "%~dp0\..\which\bin\which" %*
|
||||
) ELSE (
|
||||
@SETLOCAL
|
||||
@SET PATHEXT=%PATHEXT:;.JS;=;%
|
||||
node "%~dp0\..\which\bin\which" %*
|
||||
)
|
236
node_modules/accepts/HISTORY.md
generated
vendored
Normal file
@ -0,0 +1,236 @@
|
||||
1.3.7 / 2019-04-29
|
||||
==================
|
||||
|
||||
* deps: negotiator@0.6.2
|
||||
- Fix sorting charset, encoding, and language with extra parameters
|
||||
|
||||
1.3.6 / 2019-04-28
|
||||
==================
|
||||
|
||||
* deps: mime-types@~2.1.24
|
||||
- deps: mime-db@~1.40.0
|
||||
|
||||
1.3.5 / 2018-02-28
|
||||
==================
|
||||
|
||||
* deps: mime-types@~2.1.18
|
||||
- deps: mime-db@~1.33.0
|
||||
|
||||
1.3.4 / 2017-08-22
|
||||
==================
|
||||
|
||||
* deps: mime-types@~2.1.16
|
||||
- deps: mime-db@~1.29.0
|
||||
|
||||
1.3.3 / 2016-05-02
|
||||
==================
|
||||
|
||||
* deps: mime-types@~2.1.11
|
||||
- deps: mime-db@~1.23.0
|
||||
* deps: negotiator@0.6.1
|
||||
- perf: improve `Accept` parsing speed
|
||||
- perf: improve `Accept-Charset` parsing speed
|
||||
- perf: improve `Accept-Encoding` parsing speed
|
||||
- perf: improve `Accept-Language` parsing speed
|
||||
|
||||
1.3.2 / 2016-03-08
|
||||
==================
|
||||
|
||||
* deps: mime-types@~2.1.10
|
||||
- Fix extension of `application/dash+xml`
|
||||
- Update primary extension for `audio/mp4`
|
||||
- deps: mime-db@~1.22.0
|
||||
|
||||
1.3.1 / 2016-01-19
|
||||
==================
|
||||
|
||||
* deps: mime-types@~2.1.9
|
||||
- deps: mime-db@~1.21.0
|
||||
|
||||
1.3.0 / 2015-09-29
|
||||
==================
|
||||
|
||||
* deps: mime-types@~2.1.7
|
||||
- deps: mime-db@~1.19.0
|
||||
* deps: negotiator@0.6.0
|
||||
- Fix including type extensions in parameters in `Accept` parsing
|
||||
- Fix parsing `Accept` parameters with quoted equals
|
||||
- Fix parsing `Accept` parameters with quoted semicolons
|
||||
- Lazy-load modules from main entry point
|
||||
- perf: delay type concatenation until needed
|
||||
- perf: enable strict mode
|
||||
- perf: hoist regular expressions
|
||||
- perf: remove closures getting spec properties
|
||||
- perf: remove a closure from media type parsing
|
||||
- perf: remove property delete from media type parsing
|
||||
|
||||
1.2.13 / 2015-09-06
|
||||
===================
|
||||
|
||||
* deps: mime-types@~2.1.6
|
||||
- deps: mime-db@~1.18.0
|
||||
|
||||
1.2.12 / 2015-07-30
|
||||
===================
|
||||
|
||||
* deps: mime-types@~2.1.4
|
||||
- deps: mime-db@~1.16.0
|
||||
|
||||
1.2.11 / 2015-07-16
|
||||
===================
|
||||
|
||||
* deps: mime-types@~2.1.3
|
||||
- deps: mime-db@~1.15.0
|
||||
|
||||
1.2.10 / 2015-07-01
|
||||
===================
|
||||
|
||||
* deps: mime-types@~2.1.2
|
||||
- deps: mime-db@~1.14.0
|
||||
|
||||
1.2.9 / 2015-06-08
|
||||
==================
|
||||
|
||||
* deps: mime-types@~2.1.1
|
||||
- perf: fix deopt during mapping
|
||||
|
||||
1.2.8 / 2015-06-07
|
||||
==================
|
||||
|
||||
* deps: mime-types@~2.1.0
|
||||
- deps: mime-db@~1.13.0
|
||||
* perf: avoid argument reassignment & argument slice
|
||||
* perf: avoid negotiator recursive construction
|
||||
* perf: enable strict mode
|
||||
* perf: remove unnecessary bitwise operator
|
||||
|
||||
1.2.7 / 2015-05-10
|
||||
==================
|
||||
|
||||
* deps: negotiator@0.5.3
|
||||
- Fix media type parameter matching to be case-insensitive
|
||||
|
||||
1.2.6 / 2015-05-07
|
||||
==================
|
||||
|
||||
* deps: mime-types@~2.0.11
|
||||
- deps: mime-db@~1.9.1
|
||||
* deps: negotiator@0.5.2
|
||||
- Fix comparing media types with quoted values
|
||||
- Fix splitting media types with quoted commas
|
||||
|
||||
1.2.5 / 2015-03-13
|
||||
==================
|
||||
|
||||
* deps: mime-types@~2.0.10
|
||||
- deps: mime-db@~1.8.0
|
||||
|
||||
1.2.4 / 2015-02-14
|
||||
==================
|
||||
|
||||
* Support Node.js 0.6
|
||||
* deps: mime-types@~2.0.9
|
||||
- deps: mime-db@~1.7.0
|
||||
* deps: negotiator@0.5.1
|
||||
- Fix preference sorting to be stable for long acceptable lists
|
||||
|
||||
1.2.3 / 2015-01-31
|
||||
==================
|
||||
|
||||
* deps: mime-types@~2.0.8
|
||||
- deps: mime-db@~1.6.0
|
||||
|
||||
1.2.2 / 2014-12-30
|
||||
==================
|
||||
|
||||
* deps: mime-types@~2.0.7
|
||||
- deps: mime-db@~1.5.0
|
||||
|
||||
1.2.1 / 2014-12-30
|
||||
==================
|
||||
|
||||
* deps: mime-types@~2.0.5
|
||||
- deps: mime-db@~1.3.1
|
||||
|
||||
1.2.0 / 2014-12-19
|
||||
==================
|
||||
|
||||
* deps: negotiator@0.5.0
|
||||
- Fix list return order when large accepted list
|
||||
- Fix missing identity encoding when q=0 exists
|
||||
- Remove dynamic building of Negotiator class
|
||||
|
||||
1.1.4 / 2014-12-10
|
||||
==================
|
||||
|
||||
* deps: mime-types@~2.0.4
|
||||
- deps: mime-db@~1.3.0
|
||||
|
||||
1.1.3 / 2014-11-09
|
||||
==================
|
||||
|
||||
* deps: mime-types@~2.0.3
|
||||
- deps: mime-db@~1.2.0
|
||||
|
||||
1.1.2 / 2014-10-14
|
||||
==================
|
||||
|
||||
* deps: negotiator@0.4.9
|
||||
- Fix error when media type has invalid parameter
|
||||
|
||||
1.1.1 / 2014-09-28
|
||||
==================
|
||||
|
||||
* deps: mime-types@~2.0.2
|
||||
- deps: mime-db@~1.1.0
|
||||
* deps: negotiator@0.4.8
|
||||
- Fix all negotiations to be case-insensitive
|
||||
- Stable sort preferences of same quality according to client order
|
||||
|
||||
1.1.0 / 2014-09-02
|
||||
==================
|
||||
|
||||
* update `mime-types`
|
||||
|
||||
1.0.7 / 2014-07-04
|
||||
==================
|
||||
|
||||
* Fix wrong type returned from `type` when match after unknown extension
|
||||
|
||||
1.0.6 / 2014-06-24
|
||||
==================
|
||||
|
||||
* deps: negotiator@0.4.7
|
||||
|
||||
1.0.5 / 2014-06-20
|
||||
==================
|
||||
|
||||
* fix crash when unknown extension given
|
||||
|
||||
1.0.4 / 2014-06-19
|
||||
==================
|
||||
|
||||
* use `mime-types`
|
||||
|
||||
1.0.3 / 2014-06-11
|
||||
==================
|
||||
|
||||
* deps: negotiator@0.4.6
|
||||
- Order by specificity when quality is the same
|
||||
|
||||
1.0.2 / 2014-05-29
|
||||
==================
|
||||
|
||||
* Fix interpretation when header not in request
|
||||
* deps: pin negotiator@0.4.5
|
||||
|
||||
1.0.1 / 2014-01-18
|
||||
==================
|
||||
|
||||
* Identity encoding isn't always acceptable
|
||||
* deps: negotiator@~0.4.0
|
||||
|
||||
1.0.0 / 2013-12-27
|
||||
==================
|
||||
|
||||
* Genesis
|
23
node_modules/accepts/LICENSE
generated
vendored
Normal file
@ -0,0 +1,23 @@
|
||||
(The MIT License)
|
||||
|
||||
Copyright (c) 2014 Jonathan Ong <me@jongleberry.com>
|
||||
Copyright (c) 2015 Douglas Christopher Wilson <doug@somethingdoug.com>
|
||||
|
||||
Permission is hereby granted, free of charge, to any person obtaining
|
||||
a copy of this software and associated documentation files (the
|
||||
'Software'), to deal in the Software without restriction, including
|
||||
without limitation the rights to use, copy, modify, merge, publish,
|
||||
distribute, sublicense, and/or sell copies of the Software, and to
|
||||
permit persons to whom the Software is furnished to do so, subject to
|
||||
the following conditions:
|
||||
|
||||
The above copyright notice and this permission notice shall be
|
||||
included in all copies or substantial portions of the Software.
|
||||
|
||||
THE SOFTWARE IS PROVIDED 'AS IS', WITHOUT WARRANTY OF ANY KIND,
|
||||
EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT.
|
||||
IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY
|
||||
CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT,
|
||||
TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE
|
||||
SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
142
node_modules/accepts/README.md
generated
vendored
Normal file
@ -0,0 +1,142 @@
|
||||
# accepts
|
||||
|
||||
[![NPM Version][npm-version-image]][npm-url]
|
||||
[![NPM Downloads][npm-downloads-image]][npm-url]
|
||||
[![Node.js Version][node-version-image]][node-version-url]
|
||||
[![Build Status][travis-image]][travis-url]
|
||||
[![Test Coverage][coveralls-image]][coveralls-url]
|
||||
|
||||
Higher level content negotiation based on [negotiator](https://www.npmjs.com/package/negotiator).
|
||||
Extracted from [koa](https://www.npmjs.com/package/koa) for general use.
|
||||
|
||||
In addition to negotiator, it allows:
|
||||
|
||||
- Allows types as an array or arguments list, ie `(['text/html', 'application/json'])`
|
||||
as well as `('text/html', 'application/json')`.
|
||||
- Allows type shorthands such as `json`.
|
||||
- Returns `false` when no types match
|
||||
- Treats non-existent headers as `*`
|
||||
|
||||
## Installation
|
||||
|
||||
This is a [Node.js](https://nodejs.org/en/) module available through the
|
||||
[npm registry](https://www.npmjs.com/). Installation is done using the
|
||||
[`npm install` command](https://docs.npmjs.com/getting-started/installing-npm-packages-locally):
|
||||
|
||||
```sh
|
||||
$ npm install accepts
|
||||
```
|
||||
|
||||
## API
|
||||
|
||||
<!-- eslint-disable no-unused-vars -->
|
||||
|
||||
```js
|
||||
var accepts = require('accepts')
|
||||
```
|
||||
|
||||
### accepts(req)
|
||||
|
||||
Create a new `Accepts` object for the given `req`.
|
||||
|
||||
#### .charset(charsets)
|
||||
|
||||
Return the first accepted charset. If nothing in `charsets` is accepted,
|
||||
then `false` is returned.
|
||||
|
||||
#### .charsets()
|
||||
|
||||
Return the charsets that the request accepts, in the order of the client's
|
||||
preference (most preferred first).
|
||||
|
||||
#### .encoding(encodings)
|
||||
|
||||
Return the first accepted encoding. If nothing in `encodings` is accepted,
|
||||
then `false` is returned.
|
||||
|
||||
#### .encodings()
|
||||
|
||||
Return the encodings that the request accepts, in the order of the client's
|
||||
preference (most preferred first).
|
||||
|
||||
#### .language(languages)
|
||||
|
||||
Return the first accepted language. If nothing in `languages` is accepted,
|
||||
then `false` is returned.
|
||||
|
||||
#### .languages()
|
||||
|
||||
Return the languages that the request accepts, in the order of the client's
|
||||
preference (most preferred first).
|
||||
|
||||
#### .type(types)
|
||||
|
||||
Return the first accepted type (and it is returned as the same text as what
|
||||
appears in the `types` array). If nothing in `types` is accepted, then `false`
|
||||
is returned.
|
||||
|
||||
The `types` array can contain full MIME types or file extensions. Any value
|
||||
that is not a full MIME types is passed to `require('mime-types').lookup`.
|
||||
|
||||
#### .types()
|
||||
|
||||
Return the types that the request accepts, in the order of the client's
|
||||
preference (most preferred first).
|
||||
|
||||
## Examples
|
||||
|
||||
### Simple type negotiation
|
||||
|
||||
This simple example shows how to use `accepts` to return a different typed
|
||||
respond body based on what the client wants to accept. The server lists it's
|
||||
preferences in order and will get back the best match between the client and
|
||||
server.
|
||||
|
||||
```js
|
||||
var accepts = require('accepts')
|
||||
var http = require('http')
|
||||
|
||||
function app (req, res) {
|
||||
var accept = accepts(req)
|
||||
|
||||
// the order of this list is significant; should be server preferred order
|
||||
switch (accept.type(['json', 'html'])) {
|
||||
case 'json':
|
||||
res.setHeader('Content-Type', 'application/json')
|
||||
res.write('{"hello":"world!"}')
|
||||
break
|
||||
case 'html':
|
||||
res.setHeader('Content-Type', 'text/html')
|
||||
res.write('<b>hello, world!</b>')
|
||||
break
|
||||
default:
|
||||
// the fallback is text/plain, so no need to specify it above
|
||||
res.setHeader('Content-Type', 'text/plain')
|
||||
res.write('hello, world!')
|
||||
break
|
||||
}
|
||||
|
||||
res.end()
|
||||
}
|
||||
|
||||
http.createServer(app).listen(3000)
|
||||
```
|
||||
|
||||
You can test this out with the cURL program:
|
||||
```sh
|
||||
curl -I -H'Accept: text/html' http://localhost:3000/
|
||||
```
|
||||
|
||||
## License
|
||||
|
||||
[MIT](LICENSE)
|
||||
|
||||
[coveralls-image]: https://badgen.net/coveralls/c/github/jshttp/accepts/master
|
||||
[coveralls-url]: https://coveralls.io/r/jshttp/accepts?branch=master
|
||||
[node-version-image]: https://badgen.net/npm/node/accepts
|
||||
[node-version-url]: https://nodejs.org/en/download
|
||||
[npm-downloads-image]: https://badgen.net/npm/dm/accepts
|
||||
[npm-url]: https://npmjs.org/package/accepts
|
||||
[npm-version-image]: https://badgen.net/npm/v/accepts
|
||||
[travis-image]: https://badgen.net/travis/jshttp/accepts/master
|
||||
[travis-url]: https://travis-ci.org/jshttp/accepts
|
238
node_modules/accepts/index.js
generated
vendored
Normal file
@ -0,0 +1,238 @@
|
||||
/*!
|
||||
* accepts
|
||||
* Copyright(c) 2014 Jonathan Ong
|
||||
* Copyright(c) 2015 Douglas Christopher Wilson
|
||||
* MIT Licensed
|
||||
*/
|
||||
|
||||
'use strict'
|
||||
|
||||
/**
|
||||
* Module dependencies.
|
||||
* @private
|
||||
*/
|
||||
|
||||
var Negotiator = require('negotiator')
|
||||
var mime = require('mime-types')
|
||||
|
||||
/**
|
||||
* Module exports.
|
||||
* @public
|
||||
*/
|
||||
|
||||
module.exports = Accepts
|
||||
|
||||
/**
|
||||
* Create a new Accepts object for the given req.
|
||||
*
|
||||
* @param {object} req
|
||||
* @public
|
||||
*/
|
||||
|
||||
function Accepts (req) {
|
||||
if (!(this instanceof Accepts)) {
|
||||
return new Accepts(req)
|
||||
}
|
||||
|
||||
this.headers = req.headers
|
||||
this.negotiator = new Negotiator(req)
|
||||
}
|
||||
|
||||
/**
|
||||
* Check if the given `type(s)` is acceptable, returning
|
||||
* the best match when true, otherwise `undefined`, in which
|
||||
* case you should respond with 406 "Not Acceptable".
|
||||
*
|
||||
* The `type` value may be a single mime type string
|
||||
* such as "application/json", the extension name
|
||||
* such as "json" or an array `["json", "html", "text/plain"]`. When a list
|
||||
* or array is given the _best_ match, if any is returned.
|
||||
*
|
||||
* Examples:
|
||||
*
|
||||
* // Accept: text/html
|
||||
* this.types('html');
|
||||
* // => "html"
|
||||
*
|
||||
* // Accept: text/*, application/json
|
||||
* this.types('html');
|
||||
* // => "html"
|
||||
* this.types('text/html');
|
||||
* // => "text/html"
|
||||
* this.types('json', 'text');
|
||||
* // => "json"
|
||||
* this.types('application/json');
|
||||
* // => "application/json"
|
||||
*
|
||||
* // Accept: text/*, application/json
|
||||
* this.types('image/png');
|
||||
* this.types('png');
|
||||
* // => undefined
|
||||
*
|
||||
* // Accept: text/*;q=.5, application/json
|
||||
* this.types(['html', 'json']);
|
||||
* this.types('html', 'json');
|
||||
* // => "json"
|
||||
*
|
||||
* @param {String|Array} types...
|
||||
* @return {String|Array|Boolean}
|
||||
* @public
|
||||
*/
|
||||
|
||||
Accepts.prototype.type =
|
||||
Accepts.prototype.types = function (types_) {
|
||||
var types = types_
|
||||
|
||||
// support flattened arguments
|
||||
if (types && !Array.isArray(types)) {
|
||||
types = new Array(arguments.length)
|
||||
for (var i = 0; i < types.length; i++) {
|
||||
types[i] = arguments[i]
|
||||
}
|
||||
}
|
||||
|
||||
// no types, return all requested types
|
||||
if (!types || types.length === 0) {
|
||||
return this.negotiator.mediaTypes()
|
||||
}
|
||||
|
||||
// no accept header, return first given type
|
||||
if (!this.headers.accept) {
|
||||
return types[0]
|
||||
}
|
||||
|
||||
var mimes = types.map(extToMime)
|
||||
var accepts = this.negotiator.mediaTypes(mimes.filter(validMime))
|
||||
var first = accepts[0]
|
||||
|
||||
return first
|
||||
? types[mimes.indexOf(first)]
|
||||
: false
|
||||
}
|
||||
|
||||
/**
|
||||
* Return accepted encodings or best fit based on `encodings`.
|
||||
*
|
||||
* Given `Accept-Encoding: gzip, deflate`
|
||||
* an array sorted by quality is returned:
|
||||
*
|
||||
* ['gzip', 'deflate']
|
||||
*
|
||||
* @param {String|Array} encodings...
|
||||
* @return {String|Array}
|
||||
* @public
|
||||
*/
|
||||
|
||||
Accepts.prototype.encoding =
|
||||
Accepts.prototype.encodings = function (encodings_) {
|
||||
var encodings = encodings_
|
||||
|
||||
// support flattened arguments
|
||||
if (encodings && !Array.isArray(encodings)) {
|
||||
encodings = new Array(arguments.length)
|
||||
for (var i = 0; i < encodings.length; i++) {
|
||||
encodings[i] = arguments[i]
|
||||
}
|
||||
}
|
||||
|
||||
// no encodings, return all requested encodings
|
||||
if (!encodings || encodings.length === 0) {
|
||||
return this.negotiator.encodings()
|
||||
}
|
||||
|
||||
return this.negotiator.encodings(encodings)[0] || false
|
||||
}
|
||||
|
||||
/**
|
||||
* Return accepted charsets or best fit based on `charsets`.
|
||||
*
|
||||
* Given `Accept-Charset: utf-8, iso-8859-1;q=0.2, utf-7;q=0.5`
|
||||
* an array sorted by quality is returned:
|
||||
*
|
||||
* ['utf-8', 'utf-7', 'iso-8859-1']
|
||||
*
|
||||
* @param {String|Array} charsets...
|
||||
* @return {String|Array}
|
||||
* @public
|
||||
*/
|
||||
|
||||
Accepts.prototype.charset =
|
||||
Accepts.prototype.charsets = function (charsets_) {
|
||||
var charsets = charsets_
|
||||
|
||||
// support flattened arguments
|
||||
if (charsets && !Array.isArray(charsets)) {
|
||||
charsets = new Array(arguments.length)
|
||||
for (var i = 0; i < charsets.length; i++) {
|
||||
charsets[i] = arguments[i]
|
||||
}
|
||||
}
|
||||
|
||||
// no charsets, return all requested charsets
|
||||
if (!charsets || charsets.length === 0) {
|
||||
return this.negotiator.charsets()
|
||||
}
|
||||
|
||||
return this.negotiator.charsets(charsets)[0] || false
|
||||
}
|
||||
|
||||
/**
|
||||
* Return accepted languages or best fit based on `langs`.
|
||||
*
|
||||
* Given `Accept-Language: en;q=0.8, es, pt`
|
||||
* an array sorted by quality is returned:
|
||||
*
|
||||
* ['es', 'pt', 'en']
|
||||
*
|
||||
* @param {String|Array} langs...
|
||||
* @return {Array|String}
|
||||
* @public
|
||||
*/
|
||||
|
||||
Accepts.prototype.lang =
|
||||
Accepts.prototype.langs =
|
||||
Accepts.prototype.language =
|
||||
Accepts.prototype.languages = function (languages_) {
|
||||
var languages = languages_
|
||||
|
||||
// support flattened arguments
|
||||
if (languages && !Array.isArray(languages)) {
|
||||
languages = new Array(arguments.length)
|
||||
for (var i = 0; i < languages.length; i++) {
|
||||
languages[i] = arguments[i]
|
||||
}
|
||||
}
|
||||
|
||||
// no languages, return all requested languages
|
||||
if (!languages || languages.length === 0) {
|
||||
return this.negotiator.languages()
|
||||
}
|
||||
|
||||
return this.negotiator.languages(languages)[0] || false
|
||||
}
|
||||
|
||||
/**
|
||||
* Convert extnames to mime.
|
||||
*
|
||||
* @param {String} type
|
||||
* @return {String}
|
||||
* @private
|
||||
*/
|
||||
|
||||
function extToMime (type) {
|
||||
return type.indexOf('/') === -1
|
||||
? mime.lookup(type)
|
||||
: type
|
||||
}
|
||||
|
||||
/**
|
||||
* Check if mime is valid.
|
||||
*
|
||||
* @param {String} type
|
||||
* @return {String}
|
||||
* @private
|
||||
*/
|
||||
|
||||
function validMime (type) {
|
||||
return typeof type === 'string'
|
||||
}
|
86
node_modules/accepts/package.json
generated
vendored
Normal file
@ -0,0 +1,86 @@
|
||||
{
|
||||
"_from": "accepts@~1.3.4",
|
||||
"_id": "accepts@1.3.7",
|
||||
"_inBundle": false,
|
||||
"_integrity": "sha512-Il80Qs2WjYlJIBNzNkK6KYqlVMTbZLXgHx2oT0pU/fjRHyEp+PEfEPY0R3WCwAGVOtauxh1hOxNgIf5bv7dQpA==",
|
||||
"_location": "/accepts",
|
||||
"_phantomChildren": {},
|
||||
"_requested": {
|
||||
"type": "range",
|
||||
"registry": true,
|
||||
"raw": "accepts@~1.3.4",
|
||||
"name": "accepts",
|
||||
"escapedName": "accepts",
|
||||
"rawSpec": "~1.3.4",
|
||||
"saveSpec": null,
|
||||
"fetchSpec": "~1.3.4"
|
||||
},
|
||||
"_requiredBy": [
|
||||
"/serve-index"
|
||||
],
|
||||
"_resolved": "https://registry.npmjs.org/accepts/-/accepts-1.3.7.tgz",
|
||||
"_shasum": "531bc726517a3b2b41f850021c6cc15eaab507cd",
|
||||
"_spec": "accepts@~1.3.4",
|
||||
"_where": "C:\\Users\\User\\Documents\\Git\\asdf-games\\node_modules\\serve-index",
|
||||
"bugs": {
|
||||
"url": "https://github.com/jshttp/accepts/issues"
|
||||
},
|
||||
"bundleDependencies": false,
|
||||
"contributors": [
|
||||
{
|
||||
"name": "Douglas Christopher Wilson",
|
||||
"email": "doug@somethingdoug.com"
|
||||
},
|
||||
{
|
||||
"name": "Jonathan Ong",
|
||||
"email": "me@jongleberry.com",
|
||||
"url": "http://jongleberry.com"
|
||||
}
|
||||
],
|
||||
"dependencies": {
|
||||
"mime-types": "~2.1.24",
|
||||
"negotiator": "0.6.2"
|
||||
},
|
||||
"deprecated": false,
|
||||
"description": "Higher-level content negotiation",
|
||||
"devDependencies": {
|
||||
"deep-equal": "1.0.1",
|
||||
"eslint": "5.16.0",
|
||||
"eslint-config-standard": "12.0.0",
|
||||
"eslint-plugin-import": "2.17.2",
|
||||
"eslint-plugin-markdown": "1.0.0",
|
||||
"eslint-plugin-node": "8.0.1",
|
||||
"eslint-plugin-promise": "4.1.1",
|
||||
"eslint-plugin-standard": "4.0.0",
|
||||
"mocha": "6.1.4",
|
||||
"nyc": "14.0.0"
|
||||
},
|
||||
"engines": {
|
||||
"node": ">= 0.6"
|
||||
},
|
||||
"files": [
|
||||
"LICENSE",
|
||||
"HISTORY.md",
|
||||
"index.js"
|
||||
],
|
||||
"homepage": "https://github.com/jshttp/accepts#readme",
|
||||
"keywords": [
|
||||
"content",
|
||||
"negotiation",
|
||||
"accept",
|
||||
"accepts"
|
||||
],
|
||||
"license": "MIT",
|
||||
"name": "accepts",
|
||||
"repository": {
|
||||
"type": "git",
|
||||
"url": "git+https://github.com/jshttp/accepts.git"
|
||||
},
|
||||
"scripts": {
|
||||
"lint": "eslint --plugin markdown --ext js,md .",
|
||||
"test": "mocha --reporter spec --check-leaks --bail test/",
|
||||
"test-cov": "nyc --reporter=html --reporter=text npm test",
|
||||
"test-travis": "nyc --reporter=text npm test"
|
||||
},
|
||||
"version": "1.3.7"
|
||||
}
|
4
node_modules/ansi-regex/index.js
generated
vendored
Normal file
@ -0,0 +1,4 @@
|
||||
'use strict';
|
||||
module.exports = function () {
|
||||
return /\u001b\[(?:[0-9]{1,3}(?:;[0-9]{1,3})*)?[m|K]/g;
|
||||
};
|
84
node_modules/ansi-regex/package.json
generated
vendored
Normal file
@ -0,0 +1,84 @@
|
||||
{
|
||||
"_from": "ansi-regex@^0.2.0",
|
||||
"_id": "ansi-regex@0.2.1",
|
||||
"_inBundle": false,
|
||||
"_integrity": "sha1-DY6UaWej2BQ/k+JOKYUl/BsiNfk=",
|
||||
"_location": "/ansi-regex",
|
||||
"_phantomChildren": {},
|
||||
"_requested": {
|
||||
"type": "range",
|
||||
"registry": true,
|
||||
"raw": "ansi-regex@^0.2.0",
|
||||
"name": "ansi-regex",
|
||||
"escapedName": "ansi-regex",
|
||||
"rawSpec": "^0.2.0",
|
||||
"saveSpec": null,
|
||||
"fetchSpec": "^0.2.0"
|
||||
},
|
||||
"_requiredBy": [
|
||||
"/chalk/strip-ansi",
|
||||
"/has-ansi"
|
||||
],
|
||||
"_resolved": "https://registry.npmjs.org/ansi-regex/-/ansi-regex-0.2.1.tgz",
|
||||
"_shasum": "0d8e946967a3d8143f93e24e298525fc1b2235f9",
|
||||
"_spec": "ansi-regex@^0.2.0",
|
||||
"_where": "C:\\Users\\User\\Documents\\Git\\asdf-games\\node_modules\\has-ansi",
|
||||
"author": {
|
||||
"name": "Sindre Sorhus",
|
||||
"email": "sindresorhus@gmail.com",
|
||||
"url": "http://sindresorhus.com"
|
||||
},
|
||||
"bugs": {
|
||||
"url": "https://github.com/sindresorhus/ansi-regex/issues"
|
||||
},
|
||||
"bundleDependencies": false,
|
||||
"deprecated": false,
|
||||
"description": "Regular expression for matching ANSI escape codes",
|
||||
"devDependencies": {
|
||||
"mocha": "*"
|
||||
},
|
||||
"engines": {
|
||||
"node": ">=0.10.0"
|
||||
},
|
||||
"files": [
|
||||
"index.js"
|
||||
],
|
||||
"homepage": "https://github.com/sindresorhus/ansi-regex#readme",
|
||||
"keywords": [
|
||||
"ansi",
|
||||
"styles",
|
||||
"color",
|
||||
"colour",
|
||||
"colors",
|
||||
"terminal",
|
||||
"console",
|
||||
"cli",
|
||||
"string",
|
||||
"tty",
|
||||
"escape",
|
||||
"formatting",
|
||||
"rgb",
|
||||
"256",
|
||||
"shell",
|
||||
"xterm",
|
||||
"command-line",
|
||||
"text",
|
||||
"regex",
|
||||
"regexp",
|
||||
"re",
|
||||
"match",
|
||||
"test",
|
||||
"find",
|
||||
"pattern"
|
||||
],
|
||||
"license": "MIT",
|
||||
"name": "ansi-regex",
|
||||
"repository": {
|
||||
"type": "git",
|
||||
"url": "git+https://github.com/sindresorhus/ansi-regex.git"
|
||||
},
|
||||
"scripts": {
|
||||
"test": "mocha"
|
||||
},
|
||||
"version": "0.2.1"
|
||||
}
|
33
node_modules/ansi-regex/readme.md
generated
vendored
Normal file
@ -0,0 +1,33 @@
|
||||
# ansi-regex [](https://travis-ci.org/sindresorhus/ansi-regex)
|
||||
|
||||
> Regular expression for matching [ANSI escape codes](http://en.wikipedia.org/wiki/ANSI_escape_code)
|
||||
|
||||
|
||||
## Install
|
||||
|
||||
```sh
|
||||
$ npm install --save ansi-regex
|
||||
```
|
||||
|
||||
|
||||
## Usage
|
||||
|
||||
```js
|
||||
var ansiRegex = require('ansi-regex');
|
||||
|
||||
ansiRegex().test('\u001b[4mcake\u001b[0m');
|
||||
//=> true
|
||||
|
||||
ansiRegex().test('cake');
|
||||
//=> false
|
||||
|
||||
'\u001b[4mcake\u001b[0m'.match(ansiRegex());
|
||||
//=> ['\u001b[4m', '\u001b[0m']
|
||||
```
|
||||
|
||||
*It's a function so you can create multiple instances. Regexes with the global flag will have the `.lastIndex` property changed for each call to methods on the instance. Therefore reusing the instance with multiple calls will not work as expected for `.test()`.*
|
||||
|
||||
|
||||
## License
|
||||
|
||||
MIT © [Sindre Sorhus](http://sindresorhus.com)
|
40
node_modules/ansi-styles/index.js
generated
vendored
Normal file
@ -0,0 +1,40 @@
|
||||
'use strict';
|
||||
var styles = module.exports;
|
||||
|
||||
var codes = {
|
||||
reset: [0, 0],
|
||||
|
||||
bold: [1, 22], // 21 isn't widely supported and 22 does the same thing
|
||||
dim: [2, 22],
|
||||
italic: [3, 23],
|
||||
underline: [4, 24],
|
||||
inverse: [7, 27],
|
||||
hidden: [8, 28],
|
||||
strikethrough: [9, 29],
|
||||
|
||||
black: [30, 39],
|
||||
red: [31, 39],
|
||||
green: [32, 39],
|
||||
yellow: [33, 39],
|
||||
blue: [34, 39],
|
||||
magenta: [35, 39],
|
||||
cyan: [36, 39],
|
||||
white: [37, 39],
|
||||
gray: [90, 39],
|
||||
|
||||
bgBlack: [40, 49],
|
||||
bgRed: [41, 49],
|
||||
bgGreen: [42, 49],
|
||||
bgYellow: [43, 49],
|
||||
bgBlue: [44, 49],
|
||||
bgMagenta: [45, 49],
|
||||
bgCyan: [46, 49],
|
||||
bgWhite: [47, 49]
|
||||
};
|
||||
|
||||
Object.keys(codes).forEach(function (key) {
|
||||
var val = codes[key];
|
||||
var style = styles[key] = {};
|
||||
style.open = '\u001b[' + val[0] + 'm';
|
||||
style.close = '\u001b[' + val[1] + 'm';
|
||||
});
|
78
node_modules/ansi-styles/package.json
generated
vendored
Normal file
@ -0,0 +1,78 @@
|
||||
{
|
||||
"_from": "ansi-styles@^1.1.0",
|
||||
"_id": "ansi-styles@1.1.0",
|
||||
"_inBundle": false,
|
||||
"_integrity": "sha1-6uy/Zs1waIJ2Cy9GkVgrj1XXp94=",
|
||||
"_location": "/ansi-styles",
|
||||
"_phantomChildren": {},
|
||||
"_requested": {
|
||||
"type": "range",
|
||||
"registry": true,
|
||||
"raw": "ansi-styles@^1.1.0",
|
||||
"name": "ansi-styles",
|
||||
"escapedName": "ansi-styles",
|
||||
"rawSpec": "^1.1.0",
|
||||
"saveSpec": null,
|
||||
"fetchSpec": "^1.1.0"
|
||||
},
|
||||
"_requiredBy": [
|
||||
"/chalk"
|
||||
],
|
||||
"_resolved": "https://registry.npmjs.org/ansi-styles/-/ansi-styles-1.1.0.tgz",
|
||||
"_shasum": "eaecbf66cd706882760b2f4691582b8f55d7a7de",
|
||||
"_spec": "ansi-styles@^1.1.0",
|
||||
"_where": "C:\\Users\\User\\Documents\\Git\\asdf-games\\node_modules\\chalk",
|
||||
"author": {
|
||||
"name": "Sindre Sorhus",
|
||||
"email": "sindresorhus@gmail.com",
|
||||
"url": "http://sindresorhus.com"
|
||||
},
|
||||
"bugs": {
|
||||
"url": "https://github.com/sindresorhus/ansi-styles/issues"
|
||||
},
|
||||
"bundleDependencies": false,
|
||||
"deprecated": false,
|
||||
"description": "ANSI escape codes for styling strings in the terminal",
|
||||
"devDependencies": {
|
||||
"mocha": "*"
|
||||
},
|
||||
"engines": {
|
||||
"node": ">=0.10.0"
|
||||
},
|
||||
"files": [
|
||||
"index.js"
|
||||
],
|
||||
"homepage": "https://github.com/sindresorhus/ansi-styles#readme",
|
||||
"keywords": [
|
||||
"ansi",
|
||||
"styles",
|
||||
"color",
|
||||
"colour",
|
||||
"colors",
|
||||
"terminal",
|
||||
"console",
|
||||
"cli",
|
||||
"string",
|
||||
"tty",
|
||||
"escape",
|
||||
"formatting",
|
||||
"rgb",
|
||||
"256",
|
||||
"shell",
|
||||
"xterm",
|
||||
"log",
|
||||
"logging",
|
||||
"command-line",
|
||||
"text"
|
||||
],
|
||||
"license": "MIT",
|
||||
"name": "ansi-styles",
|
||||
"repository": {
|
||||
"type": "git",
|
||||
"url": "git+https://github.com/sindresorhus/ansi-styles.git"
|
||||
},
|
||||
"scripts": {
|
||||
"test": "mocha"
|
||||
},
|
||||
"version": "1.1.0"
|
||||
}
|
70
node_modules/ansi-styles/readme.md
generated
vendored
Normal file
@ -0,0 +1,70 @@
|
||||
# ansi-styles [](https://travis-ci.org/sindresorhus/ansi-styles)
|
||||
|
||||
> [ANSI escape codes](http://en.wikipedia.org/wiki/ANSI_escape_code#Colors_and_Styles) for styling strings in the terminal
|
||||
|
||||
You probably want the higher-level [chalk](https://github.com/sindresorhus/chalk) module for styling your strings.
|
||||
|
||||

|
||||
|
||||
|
||||
## Install
|
||||
|
||||
```sh
|
||||
$ npm install --save ansi-styles
|
||||
```
|
||||
|
||||
|
||||
## Usage
|
||||
|
||||
```js
|
||||
var ansi = require('ansi-styles');
|
||||
|
||||
console.log(ansi.green.open + 'Hello world!' + ansi.green.close);
|
||||
```
|
||||
|
||||
|
||||
## API
|
||||
|
||||
Each style has an `open` and `close` property.
|
||||
|
||||
|
||||
## Styles
|
||||
|
||||
### General
|
||||
|
||||
- `reset`
|
||||
- `bold`
|
||||
- `dim`
|
||||
- `italic` *(not widely supported)*
|
||||
- `underline`
|
||||
- `inverse`
|
||||
- `hidden`
|
||||
- `strikethrough` *(not widely supported)*
|
||||
|
||||
### Text colors
|
||||
|
||||
- `black`
|
||||
- `red`
|
||||
- `green`
|
||||
- `yellow`
|
||||
- `blue`
|
||||
- `magenta`
|
||||
- `cyan`
|
||||
- `white`
|
||||
- `gray`
|
||||
|
||||
### Background colors
|
||||
|
||||
- `bgBlack`
|
||||
- `bgRed`
|
||||
- `bgGreen`
|
||||
- `bgYellow`
|
||||
- `bgBlue`
|
||||
- `bgMagenta`
|
||||
- `bgCyan`
|
||||
- `bgWhite`
|
||||
|
||||
|
||||
## License
|
||||
|
||||
MIT © [Sindre Sorhus](http://sindresorhus.com)
|
15
node_modules/anymatch/LICENSE
generated
vendored
Normal file
@ -0,0 +1,15 @@
|
||||
The ISC License
|
||||
|
||||
Copyright (c) 2014 Elan Shanker
|
||||
|
||||
Permission to use, copy, modify, and/or distribute this software for any
|
||||
purpose with or without fee is hereby granted, provided that the above
|
||||
copyright notice and this permission notice appear in all copies.
|
||||
|
||||
THE SOFTWARE IS PROVIDED "AS IS" AND THE AUTHOR DISCLAIMS ALL WARRANTIES
|
||||
WITH REGARD TO THIS SOFTWARE INCLUDING ALL IMPLIED WARRANTIES OF
|
||||
MERCHANTABILITY AND FITNESS. IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR
|
||||
ANY SPECIAL, DIRECT, INDIRECT, OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES
|
||||
WHATSOEVER RESULTING FROM LOSS OF USE, DATA OR PROFITS, WHETHER IN AN
|
||||
ACTION OF CONTRACT, NEGLIGENCE OR OTHER TORTIOUS ACTION, ARISING OUT OF OR
|
||||
IN CONNECTION WITH THE USE OR PERFORMANCE OF THIS SOFTWARE.
|
99
node_modules/anymatch/README.md
generated
vendored
Normal file
@ -0,0 +1,99 @@
|
||||
anymatch [](https://travis-ci.org/micromatch/anymatch) [](https://coveralls.io/r/micromatch/anymatch?branch=master)
|
||||
======
|
||||
Javascript module to match a string against a regular expression, glob, string,
|
||||
or function that takes the string as an argument and returns a truthy or falsy
|
||||
value. The matcher can also be an array of any or all of these. Useful for
|
||||
allowing a very flexible user-defined config to define things like file paths.
|
||||
|
||||
__Note: This module has Bash-parity, please be aware that Windows-style backslashes are not supported as separators. See https://github.com/micromatch/micromatch#backslashes for more information.__
|
||||
|
||||
[](https://nodei.co/npm/anymatch/)
|
||||
[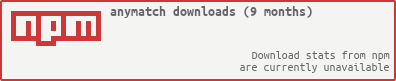](https://nodei.co/npm-dl/anymatch/)
|
||||
|
||||
Usage
|
||||
-----
|
||||
```sh
|
||||
npm install anymatch --save
|
||||
```
|
||||
|
||||
#### anymatch (matchers, testString, [returnIndex], [startIndex], [endIndex])
|
||||
* __matchers__: (_Array|String|RegExp|Function_)
|
||||
String to be directly matched, string with glob patterns, regular expression
|
||||
test, function that takes the testString as an argument and returns a truthy
|
||||
value if it should be matched, or an array of any number and mix of these types.
|
||||
* __testString__: (_String|Array_) The string to test against the matchers. If
|
||||
passed as an array, the first element of the array will be used as the
|
||||
`testString` for non-function matchers, while the entire array will be applied
|
||||
as the arguments for function matchers.
|
||||
* __returnIndex__: (_Boolean [optional]_) If true, return the array index of
|
||||
the first matcher that that testString matched, or -1 if no match, instead of a
|
||||
boolean result.
|
||||
* __startIndex, endIndex__: (_Integer [optional]_) Can be used to define a
|
||||
subset out of the array of provided matchers to test against. Can be useful
|
||||
with bound matcher functions (see below). When used with `returnIndex = true`
|
||||
preserves original indexing. Behaves the same as `Array.prototype.slice` (i.e.
|
||||
includes array members up to, but not including endIndex).
|
||||
|
||||
```js
|
||||
var anymatch = require('anymatch');
|
||||
|
||||
var matchers = [
|
||||
'path/to/file.js',
|
||||
'path/anyjs/**/*.js',
|
||||
/foo\.js$/,
|
||||
function (string) {
|
||||
return string.indexOf('bar') !== -1 && string.length > 10
|
||||
}
|
||||
];
|
||||
|
||||
anymatch(matchers, 'path/to/file.js'); // true
|
||||
anymatch(matchers, 'path/anyjs/baz.js'); // true
|
||||
anymatch(matchers, 'path/to/foo.js'); // true
|
||||
anymatch(matchers, 'path/to/bar.js'); // true
|
||||
anymatch(matchers, 'bar.js'); // false
|
||||
|
||||
// returnIndex = true
|
||||
anymatch(matchers, 'foo.js', true); // 2
|
||||
anymatch(matchers, 'path/anyjs/foo.js', true); // 1
|
||||
|
||||
// skip matchers
|
||||
anymatch(matchers, 'path/to/file.js', false, 1); // false
|
||||
anymatch(matchers, 'path/anyjs/foo.js', true, 2, 3); // 2
|
||||
anymatch(matchers, 'path/to/bar.js', true, 0, 3); // -1
|
||||
|
||||
// using globs to match directories and their children
|
||||
anymatch('node_modules', 'node_modules'); // true
|
||||
anymatch('node_modules', 'node_modules/somelib/index.js'); // false
|
||||
anymatch('node_modules/**', 'node_modules/somelib/index.js'); // true
|
||||
anymatch('node_modules/**', '/absolute/path/to/node_modules/somelib/index.js'); // false
|
||||
anymatch('**/node_modules/**', '/absolute/path/to/node_modules/somelib/index.js'); // true
|
||||
```
|
||||
|
||||
#### anymatch (matchers)
|
||||
You can also pass in only your matcher(s) to get a curried function that has
|
||||
already been bound to the provided matching criteria. This can be used as an
|
||||
`Array.prototype.filter` callback.
|
||||
|
||||
```js
|
||||
var matcher = anymatch(matchers);
|
||||
|
||||
matcher('path/to/file.js'); // true
|
||||
matcher('path/anyjs/baz.js', true); // 1
|
||||
matcher('path/anyjs/baz.js', true, 2); // -1
|
||||
|
||||
['foo.js', 'bar.js'].filter(matcher); // ['foo.js']
|
||||
```
|
||||
|
||||
Change Log
|
||||
----------
|
||||
[See release notes page on GitHub](https://github.com/micromatch/anymatch/releases)
|
||||
|
||||
NOTE: As of v2.0.0, [micromatch](https://github.com/jonschlinkert/micromatch) moves away from minimatch-parity and inline with Bash. This includes handling backslashes differently (see https://github.com/micromatch/micromatch#backslashes for more information).
|
||||
|
||||
NOTE: As of v1.2.0, anymatch uses [micromatch](https://github.com/jonschlinkert/micromatch)
|
||||
for glob pattern matching. Issues with glob pattern matching should be
|
||||
reported directly to the [micromatch issue tracker](https://github.com/jonschlinkert/micromatch/issues).
|
||||
|
||||
License
|
||||
-------
|
||||
[ISC](https://raw.github.com/micromatch/anymatch/master/LICENSE)
|
67
node_modules/anymatch/index.js
generated
vendored
Normal file
@ -0,0 +1,67 @@
|
||||
'use strict';
|
||||
|
||||
var micromatch = require('micromatch');
|
||||
var normalize = require('normalize-path');
|
||||
var path = require('path'); // required for tests.
|
||||
var arrify = function(a) { return a == null ? [] : (Array.isArray(a) ? a : [a]); };
|
||||
|
||||
var anymatch = function(criteria, value, returnIndex, startIndex, endIndex) {
|
||||
criteria = arrify(criteria);
|
||||
value = arrify(value);
|
||||
if (arguments.length === 1) {
|
||||
return anymatch.bind(null, criteria.map(function(criterion) {
|
||||
return typeof criterion === 'string' && criterion[0] !== '!' ?
|
||||
micromatch.matcher(criterion) : criterion;
|
||||
}));
|
||||
}
|
||||
startIndex = startIndex || 0;
|
||||
var string = value[0];
|
||||
var altString, altValue;
|
||||
var matched = false;
|
||||
var matchIndex = -1;
|
||||
function testCriteria(criterion, index) {
|
||||
var result;
|
||||
switch (Object.prototype.toString.call(criterion)) {
|
||||
case '[object String]':
|
||||
result = string === criterion || altString && altString === criterion;
|
||||
result = result || micromatch.isMatch(string, criterion);
|
||||
break;
|
||||
case '[object RegExp]':
|
||||
result = criterion.test(string) || altString && criterion.test(altString);
|
||||
break;
|
||||
case '[object Function]':
|
||||
result = criterion.apply(null, value);
|
||||
result = result || altValue && criterion.apply(null, altValue);
|
||||
break;
|
||||
default:
|
||||
result = false;
|
||||
}
|
||||
if (result) {
|
||||
matchIndex = index + startIndex;
|
||||
}
|
||||
return result;
|
||||
}
|
||||
var crit = criteria;
|
||||
var negGlobs = crit.reduce(function(arr, criterion, index) {
|
||||
if (typeof criterion === 'string' && criterion[0] === '!') {
|
||||
if (crit === criteria) {
|
||||
// make a copy before modifying
|
||||
crit = crit.slice();
|
||||
}
|
||||
crit[index] = null;
|
||||
arr.push(criterion.substr(1));
|
||||
}
|
||||
return arr;
|
||||
}, []);
|
||||
if (!negGlobs.length || !micromatch.any(string, negGlobs)) {
|
||||
if (path.sep === '\\' && typeof string === 'string') {
|
||||
altString = normalize(string);
|
||||
altString = altString === string ? null : altString;
|
||||
if (altString) altValue = [altString].concat(value.slice(1));
|
||||
}
|
||||
matched = crit.slice(startIndex, endIndex).some(testCriteria);
|
||||
}
|
||||
return returnIndex === true ? matchIndex : matched;
|
||||
};
|
||||
|
||||
module.exports = anymatch;
|
21
node_modules/anymatch/node_modules/normalize-path/LICENSE
generated
vendored
Normal file
@ -0,0 +1,21 @@
|
||||
The MIT License (MIT)
|
||||
|
||||
Copyright (c) 2014-2017, Jon Schlinkert
|
||||
|
||||
Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
of this software and associated documentation files (the "Software"), to deal
|
||||
in the Software without restriction, including without limitation the rights
|
||||
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
copies of the Software, and to permit persons to whom the Software is
|
||||
furnished to do so, subject to the following conditions:
|
||||
|
||||
The above copyright notice and this permission notice shall be included in
|
||||
all copies or substantial portions of the Software.
|
||||
|
||||
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
|
||||
THE SOFTWARE.
|
92
node_modules/anymatch/node_modules/normalize-path/README.md
generated
vendored
Normal file
@ -0,0 +1,92 @@
|
||||
# normalize-path [](https://www.npmjs.com/package/normalize-path) [](https://npmjs.org/package/normalize-path) [](https://npmjs.org/package/normalize-path) [](https://travis-ci.org/jonschlinkert/normalize-path)
|
||||
|
||||
> Normalize file path slashes to be unix-like forward slashes. Also condenses repeat slashes to a single slash and removes and trailing slashes unless disabled.
|
||||
|
||||
## Install
|
||||
|
||||
Install with [npm](https://www.npmjs.com/):
|
||||
|
||||
```sh
|
||||
$ npm install --save normalize-path
|
||||
```
|
||||
|
||||
## Usage
|
||||
|
||||
```js
|
||||
var normalize = require('normalize-path');
|
||||
|
||||
normalize('\\foo\\bar\\baz\\');
|
||||
//=> '/foo/bar/baz'
|
||||
|
||||
normalize('./foo/bar/baz/');
|
||||
//=> './foo/bar/baz'
|
||||
```
|
||||
|
||||
Pass `false` as the last argument to **keep** trailing slashes:
|
||||
|
||||
```js
|
||||
normalize('./foo/bar/baz/', false);
|
||||
//=> './foo/bar/baz/'
|
||||
|
||||
normalize('foo\\bar\\baz\\', false);
|
||||
//=> 'foo/bar/baz/'
|
||||
```
|
||||
|
||||
## About
|
||||
|
||||
### Related projects
|
||||
|
||||
* [contains-path](https://www.npmjs.com/package/contains-path): Return true if a file path contains the given path. | [homepage](https://github.com/jonschlinkert/contains-path "Return true if a file path contains the given path.")
|
||||
* [ends-with](https://www.npmjs.com/package/ends-with): Returns `true` if the given `string` or `array` ends with `suffix` using strict equality for… [more](https://github.com/jonschlinkert/ends-with) | [homepage](https://github.com/jonschlinkert/ends-with "Returns `true` if the given `string` or `array` ends with `suffix` using strict equality for comparisons.")
|
||||
* [is-absolute](https://www.npmjs.com/package/is-absolute): Polyfill for node.js `path.isAbolute`. Returns true if a file path is absolute. | [homepage](https://github.com/jonschlinkert/is-absolute "Polyfill for node.js `path.isAbolute`. Returns true if a file path is absolute.")
|
||||
* [is-relative](https://www.npmjs.com/package/is-relative): Returns `true` if the path appears to be relative. | [homepage](https://github.com/jonschlinkert/is-relative "Returns `true` if the path appears to be relative.")
|
||||
* [parse-filepath](https://www.npmjs.com/package/parse-filepath): Pollyfill for node.js `path.parse`, parses a filepath into an object. | [homepage](https://github.com/jonschlinkert/parse-filepath "Pollyfill for node.js `path.parse`, parses a filepath into an object.")
|
||||
* [path-ends-with](https://www.npmjs.com/package/path-ends-with): Return `true` if a file path ends with the given string/suffix. | [homepage](https://github.com/jonschlinkert/path-ends-with "Return `true` if a file path ends with the given string/suffix.")
|
||||
* [path-segments](https://www.npmjs.com/package/path-segments): Get n specific segments of a file path, e.g. first 2, last 3, etc. | [homepage](https://github.com/jonschlinkert/path-segments "Get n specific segments of a file path, e.g. first 2, last 3, etc.")
|
||||
* [rewrite-ext](https://www.npmjs.com/package/rewrite-ext): Automatically re-write the destination extension of a filepath based on the source extension. e.g… [more](https://github.com/jonschlinkert/rewrite-ext) | [homepage](https://github.com/jonschlinkert/rewrite-ext "Automatically re-write the destination extension of a filepath based on the source extension. e.g `.coffee` => `.js`. This will only rename the ext, no other path parts are modified.")
|
||||
* [unixify](https://www.npmjs.com/package/unixify): Convert Windows file paths to unix paths. | [homepage](https://github.com/jonschlinkert/unixify "Convert Windows file paths to unix paths.")
|
||||
|
||||
### Contributing
|
||||
|
||||
Pull requests and stars are always welcome. For bugs and feature requests, [please create an issue](../../issues/new).
|
||||
|
||||
### Contributors
|
||||
|
||||
| **Commits** | **Contributor** |
|
||||
| --- | --- |
|
||||
| 31 | [jonschlinkert](https://github.com/jonschlinkert) |
|
||||
| 1 | [phated](https://github.com/phated) |
|
||||
|
||||
### Building docs
|
||||
|
||||
_(This project's readme.md is generated by [verb](https://github.com/verbose/verb-generate-readme), please don't edit the readme directly. Any changes to the readme must be made in the [.verb.md](.verb.md) readme template.)_
|
||||
|
||||
To generate the readme, run the following command:
|
||||
|
||||
```sh
|
||||
$ npm install -g verbose/verb#dev verb-generate-readme && verb
|
||||
```
|
||||
|
||||
### Running tests
|
||||
|
||||
Running and reviewing unit tests is a great way to get familiarized with a library and its API. You can install dependencies and run tests with the following command:
|
||||
|
||||
```sh
|
||||
$ npm install && npm test
|
||||
```
|
||||
|
||||
### Author
|
||||
|
||||
**Jon Schlinkert**
|
||||
|
||||
* [github/jonschlinkert](https://github.com/jonschlinkert)
|
||||
* [twitter/jonschlinkert](https://twitter.com/jonschlinkert)
|
||||
|
||||
### License
|
||||
|
||||
Copyright © 2017, [Jon Schlinkert](https://github.com/jonschlinkert).
|
||||
Released under the [MIT License](LICENSE).
|
||||
|
||||
***
|
||||
|
||||
_This file was generated by [verb-generate-readme](https://github.com/verbose/verb-generate-readme), v0.4.3, on March 29, 2017._
|
19
node_modules/anymatch/node_modules/normalize-path/index.js
generated
vendored
Normal file
@ -0,0 +1,19 @@
|
||||
/*!
|
||||
* normalize-path <https://github.com/jonschlinkert/normalize-path>
|
||||
*
|
||||
* Copyright (c) 2014-2017, Jon Schlinkert.
|
||||
* Released under the MIT License.
|
||||
*/
|
||||
|
||||
var removeTrailingSeparator = require('remove-trailing-separator');
|
||||
|
||||
module.exports = function normalizePath(str, stripTrailing) {
|
||||
if (typeof str !== 'string') {
|
||||
throw new TypeError('expected a string');
|
||||
}
|
||||
str = str.replace(/[\\\/]+/g, '/');
|
||||
if (stripTrailing !== false) {
|
||||
str = removeTrailingSeparator(str);
|
||||
}
|
||||
return str;
|
||||
};
|
117
node_modules/anymatch/node_modules/normalize-path/package.json
generated
vendored
Normal file
@ -0,0 +1,117 @@
|
||||
{
|
||||
"_from": "normalize-path@^2.1.1",
|
||||
"_id": "normalize-path@2.1.1",
|
||||
"_inBundle": false,
|
||||
"_integrity": "sha1-GrKLVW4Zg2Oowab35vogE3/mrtk=",
|
||||
"_location": "/anymatch/normalize-path",
|
||||
"_phantomChildren": {},
|
||||
"_requested": {
|
||||
"type": "range",
|
||||
"registry": true,
|
||||
"raw": "normalize-path@^2.1.1",
|
||||
"name": "normalize-path",
|
||||
"escapedName": "normalize-path",
|
||||
"rawSpec": "^2.1.1",
|
||||
"saveSpec": null,
|
||||
"fetchSpec": "^2.1.1"
|
||||
},
|
||||
"_requiredBy": [
|
||||
"/anymatch"
|
||||
],
|
||||
"_resolved": "https://registry.npmjs.org/normalize-path/-/normalize-path-2.1.1.tgz",
|
||||
"_shasum": "1ab28b556e198363a8c1a6f7e6fa20137fe6aed9",
|
||||
"_spec": "normalize-path@^2.1.1",
|
||||
"_where": "C:\\Users\\User\\Documents\\Git\\asdf-games\\node_modules\\anymatch",
|
||||
"author": {
|
||||
"name": "Jon Schlinkert",
|
||||
"url": "https://github.com/jonschlinkert"
|
||||
},
|
||||
"bugs": {
|
||||
"url": "https://github.com/jonschlinkert/normalize-path/issues"
|
||||
},
|
||||
"bundleDependencies": false,
|
||||
"contributors": [
|
||||
{
|
||||
"name": "Blaine Bublitz",
|
||||
"email": "blaine.bublitz@gmail.com",
|
||||
"url": "https://twitter.com/BlaineBublitz"
|
||||
},
|
||||
{
|
||||
"name": "Jon Schlinkert",
|
||||
"email": "jon.schlinkert@sellside.com",
|
||||
"url": "http://twitter.com/jonschlinkert"
|
||||
}
|
||||
],
|
||||
"dependencies": {
|
||||
"remove-trailing-separator": "^1.0.1"
|
||||
},
|
||||
"deprecated": false,
|
||||
"description": "Normalize file path slashes to be unix-like forward slashes. Also condenses repeat slashes to a single slash and removes and trailing slashes unless disabled.",
|
||||
"devDependencies": {
|
||||
"benchmarked": "^0.1.1",
|
||||
"gulp-format-md": "^0.1.11",
|
||||
"minimist": "^1.2.0",
|
||||
"mocha": "*"
|
||||
},
|
||||
"engines": {
|
||||
"node": ">=0.10.0"
|
||||
},
|
||||
"files": [
|
||||
"index.js"
|
||||
],
|
||||
"homepage": "https://github.com/jonschlinkert/normalize-path",
|
||||
"keywords": [
|
||||
"backslash",
|
||||
"file",
|
||||
"filepath",
|
||||
"fix",
|
||||
"forward",
|
||||
"fp",
|
||||
"fs",
|
||||
"normalize",
|
||||
"path",
|
||||
"slash",
|
||||
"slashes",
|
||||
"trailing",
|
||||
"unix",
|
||||
"urix"
|
||||
],
|
||||
"license": "MIT",
|
||||
"main": "index.js",
|
||||
"name": "normalize-path",
|
||||
"repository": {
|
||||
"type": "git",
|
||||
"url": "git+https://github.com/jonschlinkert/normalize-path.git"
|
||||
},
|
||||
"scripts": {
|
||||
"test": "mocha"
|
||||
},
|
||||
"verb": {
|
||||
"related": {
|
||||
"list": [
|
||||
"contains-path",
|
||||
"ends-with",
|
||||
"is-absolute",
|
||||
"is-relative",
|
||||
"parse-filepath",
|
||||
"path-ends-with",
|
||||
"path-segments",
|
||||
"rewrite-ext",
|
||||
"unixify"
|
||||
],
|
||||
"description": "Other useful libraries for working with paths in node.js:"
|
||||
},
|
||||
"toc": false,
|
||||
"layout": "default",
|
||||
"tasks": [
|
||||
"readme"
|
||||
],
|
||||
"plugins": [
|
||||
"gulp-format-md"
|
||||
],
|
||||
"lint": {
|
||||
"reflinks": true
|
||||
}
|
||||
},
|
||||
"version": "2.1.1"
|
||||
}
|
75
node_modules/anymatch/package.json
generated
vendored
Normal file
@ -0,0 +1,75 @@
|
||||
{
|
||||
"_from": "anymatch@^2.0.0",
|
||||
"_id": "anymatch@2.0.0",
|
||||
"_inBundle": false,
|
||||
"_integrity": "sha512-5teOsQWABXHHBFP9y3skS5P3d/WfWXpv3FUpy+LorMrNYaT9pI4oLMQX7jzQ2KklNpGpWHzdCXTDT2Y3XGlZBw==",
|
||||
"_location": "/anymatch",
|
||||
"_phantomChildren": {
|
||||
"remove-trailing-separator": "1.1.0"
|
||||
},
|
||||
"_requested": {
|
||||
"type": "range",
|
||||
"registry": true,
|
||||
"raw": "anymatch@^2.0.0",
|
||||
"name": "anymatch",
|
||||
"escapedName": "anymatch",
|
||||
"rawSpec": "^2.0.0",
|
||||
"saveSpec": null,
|
||||
"fetchSpec": "^2.0.0"
|
||||
},
|
||||
"_requiredBy": [
|
||||
"/chokidar",
|
||||
"/watchify"
|
||||
],
|
||||
"_resolved": "https://registry.npmjs.org/anymatch/-/anymatch-2.0.0.tgz",
|
||||
"_shasum": "bcb24b4f37934d9aa7ac17b4adaf89e7c76ef2eb",
|
||||
"_spec": "anymatch@^2.0.0",
|
||||
"_where": "C:\\Users\\User\\Documents\\Git\\asdf-games\\node_modules\\chokidar",
|
||||
"author": {
|
||||
"name": "Elan Shanker",
|
||||
"url": "http://github.com/es128"
|
||||
},
|
||||
"bugs": {
|
||||
"url": "https://github.com/micromatch/anymatch/issues"
|
||||
},
|
||||
"bundleDependencies": false,
|
||||
"dependencies": {
|
||||
"micromatch": "^3.1.4",
|
||||
"normalize-path": "^2.1.1"
|
||||
},
|
||||
"deprecated": false,
|
||||
"description": "Matches strings against configurable strings, globs, regular expressions, and/or functions",
|
||||
"devDependencies": {
|
||||
"coveralls": "^2.7.0",
|
||||
"istanbul": "^0.4.5",
|
||||
"mocha": "^3.0.0"
|
||||
},
|
||||
"files": [
|
||||
"index.js"
|
||||
],
|
||||
"homepage": "https://github.com/micromatch/anymatch",
|
||||
"keywords": [
|
||||
"match",
|
||||
"any",
|
||||
"string",
|
||||
"file",
|
||||
"fs",
|
||||
"list",
|
||||
"glob",
|
||||
"regex",
|
||||
"regexp",
|
||||
"regular",
|
||||
"expression",
|
||||
"function"
|
||||
],
|
||||
"license": "ISC",
|
||||
"name": "anymatch",
|
||||
"repository": {
|
||||
"type": "git",
|
||||
"url": "git+https://github.com/micromatch/anymatch.git"
|
||||
},
|
||||
"scripts": {
|
||||
"test": "istanbul cover _mocha && cat ./coverage/lcov.info | coveralls"
|
||||
},
|
||||
"version": "2.0.0"
|
||||
}
|
21
node_modules/arr-diff/LICENSE
generated
vendored
Normal file
@ -0,0 +1,21 @@
|
||||
The MIT License (MIT)
|
||||
|
||||
Copyright (c) 2014-2017, Jon Schlinkert
|
||||
|
||||
Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
of this software and associated documentation files (the "Software"), to deal
|
||||
in the Software without restriction, including without limitation the rights
|
||||
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
copies of the Software, and to permit persons to whom the Software is
|
||||
furnished to do so, subject to the following conditions:
|
||||
|
||||
The above copyright notice and this permission notice shall be included in
|
||||
all copies or substantial portions of the Software.
|
||||
|
||||
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
|
||||
THE SOFTWARE.
|
130
node_modules/arr-diff/README.md
generated
vendored
Normal file
@ -0,0 +1,130 @@
|
||||
# arr-diff [](https://www.npmjs.com/package/arr-diff) [](https://npmjs.org/package/arr-diff) [](https://travis-ci.org/jonschlinkert/arr-diff)
|
||||
|
||||
> Returns an array with only the unique values from the first array, by excluding all values from additional arrays using strict equality for comparisons.
|
||||
|
||||
## Install
|
||||
|
||||
Install with [npm](https://www.npmjs.com/):
|
||||
|
||||
```sh
|
||||
$ npm install --save arr-diff
|
||||
```
|
||||
|
||||
Install with [yarn](https://yarnpkg.com):
|
||||
|
||||
```sh
|
||||
$ yarn add arr-diff
|
||||
```
|
||||
|
||||
Install with [bower](https://bower.io/)
|
||||
|
||||
```sh
|
||||
$ bower install arr-diff --save
|
||||
```
|
||||
|
||||
## Usage
|
||||
|
||||
Returns the difference between the first array and additional arrays.
|
||||
|
||||
```js
|
||||
var diff = require('arr-diff');
|
||||
|
||||
var a = ['a', 'b', 'c', 'd'];
|
||||
var b = ['b', 'c'];
|
||||
|
||||
console.log(diff(a, b))
|
||||
//=> ['a', 'd']
|
||||
```
|
||||
|
||||
## Benchmarks
|
||||
|
||||
This library versus [array-differ](https://github.com/sindresorhus/array-differ), on April 14, 2017:
|
||||
|
||||
```
|
||||
Benchmarking: (4 of 4)
|
||||
· long-dupes
|
||||
· long
|
||||
· med
|
||||
· short
|
||||
|
||||
# benchmark/fixtures/long-dupes.js (100804 bytes)
|
||||
arr-diff-3.0.0 x 822 ops/sec ±0.67% (86 runs sampled)
|
||||
arr-diff-4.0.0 x 2,141 ops/sec ±0.42% (89 runs sampled)
|
||||
array-differ x 708 ops/sec ±0.70% (89 runs sampled)
|
||||
|
||||
fastest is arr-diff-4.0.0
|
||||
|
||||
# benchmark/fixtures/long.js (94529 bytes)
|
||||
arr-diff-3.0.0 x 882 ops/sec ±0.60% (87 runs sampled)
|
||||
arr-diff-4.0.0 x 2,329 ops/sec ±0.97% (83 runs sampled)
|
||||
array-differ x 769 ops/sec ±0.61% (90 runs sampled)
|
||||
|
||||
fastest is arr-diff-4.0.0
|
||||
|
||||
# benchmark/fixtures/med.js (708 bytes)
|
||||
arr-diff-3.0.0 x 856,150 ops/sec ±0.42% (89 runs sampled)
|
||||
arr-diff-4.0.0 x 4,665,249 ops/sec ±1.06% (89 runs sampled)
|
||||
array-differ x 653,888 ops/sec ±1.02% (86 runs sampled)
|
||||
|
||||
fastest is arr-diff-4.0.0
|
||||
|
||||
# benchmark/fixtures/short.js (60 bytes)
|
||||
arr-diff-3.0.0 x 3,078,467 ops/sec ±0.77% (93 runs sampled)
|
||||
arr-diff-4.0.0 x 9,213,296 ops/sec ±0.65% (89 runs sampled)
|
||||
array-differ x 1,337,051 ops/sec ±0.91% (92 runs sampled)
|
||||
|
||||
fastest is arr-diff-4.0.0
|
||||
```
|
||||
|
||||
## About
|
||||
|
||||
### Related projects
|
||||
|
||||
* [arr-flatten](https://www.npmjs.com/package/arr-flatten): Recursively flatten an array or arrays. This is the fastest implementation of array flatten. | [homepage](https://github.com/jonschlinkert/arr-flatten "Recursively flatten an array or arrays. This is the fastest implementation of array flatten.")
|
||||
* [array-filter](https://www.npmjs.com/package/array-filter): Array#filter for older browsers. | [homepage](https://github.com/juliangruber/array-filter "Array#filter for older browsers.")
|
||||
* [array-intersection](https://www.npmjs.com/package/array-intersection): Return an array with the unique values present in _all_ given arrays using strict equality… [more](https://github.com/jonschlinkert/array-intersection) | [homepage](https://github.com/jonschlinkert/array-intersection "Return an array with the unique values present in _all_ given arrays using strict equality for comparisons.")
|
||||
|
||||
### Contributing
|
||||
|
||||
Pull requests and stars are always welcome. For bugs and feature requests, [please create an issue](../../issues/new).
|
||||
|
||||
### Contributors
|
||||
|
||||
| **Commits** | **Contributor** |
|
||||
| --- | --- |
|
||||
| 33 | [jonschlinkert](https://github.com/jonschlinkert) |
|
||||
| 2 | [paulmillr](https://github.com/paulmillr) |
|
||||
|
||||
### Building docs
|
||||
|
||||
_(This project's readme.md is generated by [verb](https://github.com/verbose/verb-generate-readme), please don't edit the readme directly. Any changes to the readme must be made in the [.verb.md](.verb.md) readme template.)_
|
||||
|
||||
To generate the readme, run the following command:
|
||||
|
||||
```sh
|
||||
$ npm install -g verbose/verb#dev verb-generate-readme && verb
|
||||
```
|
||||
|
||||
### Running tests
|
||||
|
||||
Running and reviewing unit tests is a great way to get familiarized with a library and its API. You can install dependencies and run tests with the following command:
|
||||
|
||||
```sh
|
||||
$ npm install && npm test
|
||||
```
|
||||
|
||||
### Author
|
||||
|
||||
**Jon Schlinkert**
|
||||
|
||||
* [github/jonschlinkert](https://github.com/jonschlinkert)
|
||||
* [twitter/jonschlinkert](https://twitter.com/jonschlinkert)
|
||||
|
||||
### License
|
||||
|
||||
Copyright © 2017, [Jon Schlinkert](https://github.com/jonschlinkert).
|
||||
Released under the [MIT License](LICENSE).
|
||||
|
||||
***
|
||||
|
||||
_This file was generated by [verb-generate-readme](https://github.com/verbose/verb-generate-readme), v0.5.0, on April 14, 2017._
|
47
node_modules/arr-diff/index.js
generated
vendored
Normal file
@ -0,0 +1,47 @@
|
||||
/*!
|
||||
* arr-diff <https://github.com/jonschlinkert/arr-diff>
|
||||
*
|
||||
* Copyright (c) 2014-2017, Jon Schlinkert.
|
||||
* Released under the MIT License.
|
||||
*/
|
||||
|
||||
'use strict';
|
||||
|
||||
module.exports = function diff(arr/*, arrays*/) {
|
||||
var len = arguments.length;
|
||||
var idx = 0;
|
||||
while (++idx < len) {
|
||||
arr = diffArray(arr, arguments[idx]);
|
||||
}
|
||||
return arr;
|
||||
};
|
||||
|
||||
function diffArray(one, two) {
|
||||
if (!Array.isArray(two)) {
|
||||
return one.slice();
|
||||
}
|
||||
|
||||
var tlen = two.length
|
||||
var olen = one.length;
|
||||
var idx = -1;
|
||||
var arr = [];
|
||||
|
||||
while (++idx < olen) {
|
||||
var ele = one[idx];
|
||||
|
||||
var hasEle = false;
|
||||
for (var i = 0; i < tlen; i++) {
|
||||
var val = two[i];
|
||||
|
||||
if (ele === val) {
|
||||
hasEle = true;
|
||||
break;
|
||||
}
|
||||
}
|
||||
|
||||
if (hasEle === false) {
|
||||
arr.push(ele);
|
||||
}
|
||||
}
|
||||
return arr;
|
||||
}
|
109
node_modules/arr-diff/package.json
generated
vendored
Normal file
@ -0,0 +1,109 @@
|
||||
{
|
||||
"_from": "arr-diff@^4.0.0",
|
||||
"_id": "arr-diff@4.0.0",
|
||||
"_inBundle": false,
|
||||
"_integrity": "sha1-1kYQdP6/7HHn4VI1dhoyml3HxSA=",
|
||||
"_location": "/arr-diff",
|
||||
"_phantomChildren": {},
|
||||
"_requested": {
|
||||
"type": "range",
|
||||
"registry": true,
|
||||
"raw": "arr-diff@^4.0.0",
|
||||
"name": "arr-diff",
|
||||
"escapedName": "arr-diff",
|
||||
"rawSpec": "^4.0.0",
|
||||
"saveSpec": null,
|
||||
"fetchSpec": "^4.0.0"
|
||||
},
|
||||
"_requiredBy": [
|
||||
"/micromatch",
|
||||
"/nanomatch"
|
||||
],
|
||||
"_resolved": "https://registry.npmjs.org/arr-diff/-/arr-diff-4.0.0.tgz",
|
||||
"_shasum": "d6461074febfec71e7e15235761a329a5dc7c520",
|
||||
"_spec": "arr-diff@^4.0.0",
|
||||
"_where": "C:\\Users\\User\\Documents\\Git\\asdf-games\\node_modules\\micromatch",
|
||||
"author": {
|
||||
"name": "Jon Schlinkert",
|
||||
"url": "https://github.com/jonschlinkert"
|
||||
},
|
||||
"bugs": {
|
||||
"url": "https://github.com/jonschlinkert/arr-diff/issues"
|
||||
},
|
||||
"bundleDependencies": false,
|
||||
"contributors": [
|
||||
{
|
||||
"name": "Jon Schlinkert",
|
||||
"email": "jon.schlinkert@sellside.com",
|
||||
"url": "http://twitter.com/jonschlinkert"
|
||||
},
|
||||
{
|
||||
"name": "Paul Miller",
|
||||
"email": "paul+gh@paulmillr.com",
|
||||
"url": "paulmillr.com"
|
||||
}
|
||||
],
|
||||
"dependencies": {},
|
||||
"deprecated": false,
|
||||
"description": "Returns an array with only the unique values from the first array, by excluding all values from additional arrays using strict equality for comparisons.",
|
||||
"devDependencies": {
|
||||
"ansi-bold": "^0.1.1",
|
||||
"arr-flatten": "^1.0.1",
|
||||
"array-differ": "^1.0.0",
|
||||
"benchmarked": "^0.2.4",
|
||||
"gulp-format-md": "^0.1.9",
|
||||
"minimist": "^1.2.0",
|
||||
"mocha": "^2.4.5"
|
||||
},
|
||||
"engines": {
|
||||
"node": ">=0.10.0"
|
||||
},
|
||||
"files": [
|
||||
"index.js"
|
||||
],
|
||||
"homepage": "https://github.com/jonschlinkert/arr-diff",
|
||||
"keywords": [
|
||||
"arr",
|
||||
"array",
|
||||
"array differ",
|
||||
"array-differ",
|
||||
"diff",
|
||||
"differ",
|
||||
"difference"
|
||||
],
|
||||
"license": "MIT",
|
||||
"main": "index.js",
|
||||
"name": "arr-diff",
|
||||
"repository": {
|
||||
"type": "git",
|
||||
"url": "git+https://github.com/jonschlinkert/arr-diff.git"
|
||||
},
|
||||
"scripts": {
|
||||
"test": "mocha"
|
||||
},
|
||||
"verb": {
|
||||
"toc": false,
|
||||
"layout": "default",
|
||||
"tasks": [
|
||||
"readme"
|
||||
],
|
||||
"plugins": [
|
||||
"gulp-format-md"
|
||||
],
|
||||
"related": {
|
||||
"list": [
|
||||
"arr-flatten",
|
||||
"array-filter",
|
||||
"array-intersection"
|
||||
]
|
||||
},
|
||||
"reflinks": [
|
||||
"array-differ",
|
||||
"verb"
|
||||
],
|
||||
"lint": {
|
||||
"reflinks": true
|
||||
}
|
||||
},
|
||||
"version": "4.0.0"
|
||||
}
|
21
node_modules/arr-flatten/LICENSE
generated
vendored
Normal file
@ -0,0 +1,21 @@
|
||||
The MIT License (MIT)
|
||||
|
||||
Copyright (c) 2014-2017, Jon Schlinkert.
|
||||
|
||||
Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
of this software and associated documentation files (the "Software"), to deal
|
||||
in the Software without restriction, including without limitation the rights
|
||||
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
copies of the Software, and to permit persons to whom the Software is
|
||||
furnished to do so, subject to the following conditions:
|
||||
|
||||
The above copyright notice and this permission notice shall be included in
|
||||
all copies or substantial portions of the Software.
|
||||
|
||||
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
|
||||
THE SOFTWARE.
|
86
node_modules/arr-flatten/README.md
generated
vendored
Normal file
@ -0,0 +1,86 @@
|
||||
# arr-flatten [](https://www.npmjs.com/package/arr-flatten) [](https://npmjs.org/package/arr-flatten) [](https://npmjs.org/package/arr-flatten) [](https://travis-ci.org/jonschlinkert/arr-flatten) [](https://ci.appveyor.com/project/jonschlinkert/arr-flatten)
|
||||
|
||||
> Recursively flatten an array or arrays.
|
||||
|
||||
## Install
|
||||
|
||||
Install with [npm](https://www.npmjs.com/):
|
||||
|
||||
```sh
|
||||
$ npm install --save arr-flatten
|
||||
```
|
||||
|
||||
## Install
|
||||
|
||||
Install with [bower](https://bower.io/)
|
||||
|
||||
```sh
|
||||
$ bower install arr-flatten --save
|
||||
```
|
||||
|
||||
## Usage
|
||||
|
||||
```js
|
||||
var flatten = require('arr-flatten');
|
||||
|
||||
flatten(['a', ['b', ['c']], 'd', ['e']]);
|
||||
//=> ['a', 'b', 'c', 'd', 'e']
|
||||
```
|
||||
|
||||
## Why another flatten utility?
|
||||
|
||||
I wanted the fastest implementation I could find, with implementation choices that should work for 95% of use cases, but no cruft to cover the other 5%.
|
||||
|
||||
## About
|
||||
|
||||
### Related projects
|
||||
|
||||
* [arr-filter](https://www.npmjs.com/package/arr-filter): Faster alternative to javascript's native filter method. | [homepage](https://github.com/jonschlinkert/arr-filter "Faster alternative to javascript's native filter method.")
|
||||
* [arr-union](https://www.npmjs.com/package/arr-union): Combines a list of arrays, returning a single array with unique values, using strict equality… [more](https://github.com/jonschlinkert/arr-union) | [homepage](https://github.com/jonschlinkert/arr-union "Combines a list of arrays, returning a single array with unique values, using strict equality for comparisons.")
|
||||
* [array-each](https://www.npmjs.com/package/array-each): Loop over each item in an array and call the given function on every element. | [homepage](https://github.com/jonschlinkert/array-each "Loop over each item in an array and call the given function on every element.")
|
||||
* [array-unique](https://www.npmjs.com/package/array-unique): Remove duplicate values from an array. Fastest ES5 implementation. | [homepage](https://github.com/jonschlinkert/array-unique "Remove duplicate values from an array. Fastest ES5 implementation.")
|
||||
|
||||
### Contributing
|
||||
|
||||
Pull requests and stars are always welcome. For bugs and feature requests, [please create an issue](../../issues/new).
|
||||
|
||||
### Contributors
|
||||
|
||||
| **Commits** | **Contributor** |
|
||||
| --- | --- |
|
||||
| 20 | [jonschlinkert](https://github.com/jonschlinkert) |
|
||||
| 1 | [lukeed](https://github.com/lukeed) |
|
||||
|
||||
### Building docs
|
||||
|
||||
_(This project's readme.md is generated by [verb](https://github.com/verbose/verb-generate-readme), please don't edit the readme directly. Any changes to the readme must be made in the [.verb.md](.verb.md) readme template.)_
|
||||
|
||||
To generate the readme, run the following command:
|
||||
|
||||
```sh
|
||||
$ npm install -g verbose/verb#dev verb-generate-readme && verb
|
||||
```
|
||||
|
||||
### Running tests
|
||||
|
||||
Running and reviewing unit tests is a great way to get familiarized with a library and its API. You can install dependencies and run tests with the following command:
|
||||
|
||||
```sh
|
||||
$ npm install && npm test
|
||||
```
|
||||
|
||||
### Author
|
||||
|
||||
**Jon Schlinkert**
|
||||
|
||||
* [github/jonschlinkert](https://github.com/jonschlinkert)
|
||||
* [twitter/jonschlinkert](https://twitter.com/jonschlinkert)
|
||||
|
||||
### License
|
||||
|
||||
Copyright © 2017, [Jon Schlinkert](https://github.com/jonschlinkert).
|
||||
Released under the [MIT License](LICENSE).
|
||||
|
||||
***
|
||||
|
||||
_This file was generated by [verb-generate-readme](https://github.com/verbose/verb-generate-readme), v0.6.0, on July 05, 2017._
|
22
node_modules/arr-flatten/index.js
generated
vendored
Normal file
@ -0,0 +1,22 @@
|
||||
/*!
|
||||
* arr-flatten <https://github.com/jonschlinkert/arr-flatten>
|
||||
*
|
||||
* Copyright (c) 2014-2017, Jon Schlinkert.
|
||||
* Released under the MIT License.
|
||||
*/
|
||||
|
||||
'use strict';
|
||||
|
||||
module.exports = function (arr) {
|
||||
return flat(arr, []);
|
||||
};
|
||||
|
||||
function flat(arr, res) {
|
||||
var i = 0, cur;
|
||||
var len = arr.length;
|
||||
for (; i < len; i++) {
|
||||
cur = arr[i];
|
||||
Array.isArray(cur) ? flat(cur, res) : res.push(cur);
|
||||
}
|
||||
return res;
|
||||
}
|
113
node_modules/arr-flatten/package.json
generated
vendored
Normal file
@ -0,0 +1,113 @@
|
||||
{
|
||||
"_from": "arr-flatten@^1.1.0",
|
||||
"_id": "arr-flatten@1.1.0",
|
||||
"_inBundle": false,
|
||||
"_integrity": "sha512-L3hKV5R/p5o81R7O02IGnwpDmkp6E982XhtbuwSe3O4qOtMMMtodicASA1Cny2U+aCXcNpml+m4dPsvsJ3jatg==",
|
||||
"_location": "/arr-flatten",
|
||||
"_phantomChildren": {},
|
||||
"_requested": {
|
||||
"type": "range",
|
||||
"registry": true,
|
||||
"raw": "arr-flatten@^1.1.0",
|
||||
"name": "arr-flatten",
|
||||
"escapedName": "arr-flatten",
|
||||
"rawSpec": "^1.1.0",
|
||||
"saveSpec": null,
|
||||
"fetchSpec": "^1.1.0"
|
||||
},
|
||||
"_requiredBy": [
|
||||
"/braces"
|
||||
],
|
||||
"_resolved": "https://registry.npmjs.org/arr-flatten/-/arr-flatten-1.1.0.tgz",
|
||||
"_shasum": "36048bbff4e7b47e136644316c99669ea5ae91f1",
|
||||
"_spec": "arr-flatten@^1.1.0",
|
||||
"_where": "C:\\Users\\User\\Documents\\Git\\asdf-games\\node_modules\\braces",
|
||||
"author": {
|
||||
"name": "Jon Schlinkert",
|
||||
"url": "https://github.com/jonschlinkert"
|
||||
},
|
||||
"bugs": {
|
||||
"url": "https://github.com/jonschlinkert/arr-flatten/issues"
|
||||
},
|
||||
"bundleDependencies": false,
|
||||
"contributors": [
|
||||
{
|
||||
"name": "Jon Schlinkert",
|
||||
"url": "http://twitter.com/jonschlinkert"
|
||||
},
|
||||
{
|
||||
"name": "Luke Edwards",
|
||||
"url": "https://lukeed.com"
|
||||
}
|
||||
],
|
||||
"deprecated": false,
|
||||
"description": "Recursively flatten an array or arrays.",
|
||||
"devDependencies": {
|
||||
"ansi-bold": "^0.1.1",
|
||||
"array-flatten": "^2.1.1",
|
||||
"array-slice": "^1.0.0",
|
||||
"benchmarked": "^1.0.0",
|
||||
"compute-flatten": "^1.0.0",
|
||||
"flatit": "^1.1.1",
|
||||
"flatten": "^1.0.2",
|
||||
"flatten-array": "^1.0.0",
|
||||
"glob": "^7.1.1",
|
||||
"gulp-format-md": "^0.1.12",
|
||||
"just-flatten-it": "^1.1.23",
|
||||
"lodash.flattendeep": "^4.4.0",
|
||||
"m_flattened": "^1.0.1",
|
||||
"mocha": "^3.2.0",
|
||||
"utils-flatten": "^1.0.0",
|
||||
"write": "^0.3.3"
|
||||
},
|
||||
"engines": {
|
||||
"node": ">=0.10.0"
|
||||
},
|
||||
"files": [
|
||||
"index.js"
|
||||
],
|
||||
"homepage": "https://github.com/jonschlinkert/arr-flatten",
|
||||
"keywords": [
|
||||
"arr",
|
||||
"array",
|
||||
"elements",
|
||||
"flat",
|
||||
"flatten",
|
||||
"nested",
|
||||
"recurse",
|
||||
"recursive",
|
||||
"recursively"
|
||||
],
|
||||
"license": "MIT",
|
||||
"main": "index.js",
|
||||
"name": "arr-flatten",
|
||||
"repository": {
|
||||
"type": "git",
|
||||
"url": "git+https://github.com/jonschlinkert/arr-flatten.git"
|
||||
},
|
||||
"scripts": {
|
||||
"test": "mocha"
|
||||
},
|
||||
"verb": {
|
||||
"toc": false,
|
||||
"layout": "default",
|
||||
"tasks": [
|
||||
"readme"
|
||||
],
|
||||
"plugins": [
|
||||
"gulp-format-md"
|
||||
],
|
||||
"related": {
|
||||
"list": [
|
||||
"arr-filter",
|
||||
"arr-union",
|
||||
"array-each",
|
||||
"array-unique"
|
||||
]
|
||||
},
|
||||
"lint": {
|
||||
"reflinks": true
|
||||
}
|
||||
},
|
||||
"version": "1.1.0"
|
||||
}
|
21
node_modules/arr-union/LICENSE
generated
vendored
Normal file
@ -0,0 +1,21 @@
|
||||
The MIT License (MIT)
|
||||
|
||||
Copyright (c) 2014-2016, Jon Schlinkert.
|
||||
|
||||
Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
of this software and associated documentation files (the "Software"), to deal
|
||||
in the Software without restriction, including without limitation the rights
|
||||
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
copies of the Software, and to permit persons to whom the Software is
|
||||
furnished to do so, subject to the following conditions:
|
||||
|
||||
The above copyright notice and this permission notice shall be included in
|
||||
all copies or substantial portions of the Software.
|
||||
|
||||
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
|
||||
THE SOFTWARE.
|
99
node_modules/arr-union/README.md
generated
vendored
Normal file
@ -0,0 +1,99 @@
|
||||
# arr-union [](https://www.npmjs.com/package/arr-union) [](https://travis-ci.org/jonschlinkert/arr-union)
|
||||
|
||||
> Combines a list of arrays, returning a single array with unique values, using strict equality for comparisons.
|
||||
|
||||
## Install
|
||||
|
||||
Install with [npm](https://www.npmjs.com/):
|
||||
|
||||
```sh
|
||||
$ npm i arr-union --save
|
||||
```
|
||||
|
||||
## Benchmarks
|
||||
|
||||
This library is **10-20 times faster** and more performant than [array-union](https://github.com/sindresorhus/array-union).
|
||||
|
||||
See the [benchmarks](./benchmark).
|
||||
|
||||
```sh
|
||||
#1: five-arrays
|
||||
array-union x 511,121 ops/sec ±0.80% (96 runs sampled)
|
||||
arr-union x 5,716,039 ops/sec ±0.86% (93 runs sampled)
|
||||
|
||||
#2: ten-arrays
|
||||
array-union x 245,196 ops/sec ±0.69% (94 runs sampled)
|
||||
arr-union x 1,850,786 ops/sec ±0.84% (97 runs sampled)
|
||||
|
||||
#3: two-arrays
|
||||
array-union x 563,869 ops/sec ±0.97% (94 runs sampled)
|
||||
arr-union x 9,602,852 ops/sec ±0.87% (92 runs sampled)
|
||||
```
|
||||
|
||||
## Usage
|
||||
|
||||
```js
|
||||
var union = require('arr-union');
|
||||
|
||||
union(['a'], ['b', 'c'], ['d', 'e', 'f']);
|
||||
//=> ['a', 'b', 'c', 'd', 'e', 'f']
|
||||
```
|
||||
|
||||
Returns only unique elements:
|
||||
|
||||
```js
|
||||
union(['a', 'a'], ['b', 'c']);
|
||||
//=> ['a', 'b', 'c']
|
||||
```
|
||||
|
||||
## Related projects
|
||||
|
||||
* [arr-diff](https://www.npmjs.com/package/arr-diff): Returns an array with only the unique values from the first array, by excluding all… [more](https://www.npmjs.com/package/arr-diff) | [homepage](https://github.com/jonschlinkert/arr-diff)
|
||||
* [arr-filter](https://www.npmjs.com/package/arr-filter): Faster alternative to javascript's native filter method. | [homepage](https://github.com/jonschlinkert/arr-filter)
|
||||
* [arr-flatten](https://www.npmjs.com/package/arr-flatten): Recursively flatten an array or arrays. This is the fastest implementation of array flatten. | [homepage](https://github.com/jonschlinkert/arr-flatten)
|
||||
* [arr-map](https://www.npmjs.com/package/arr-map): Faster, node.js focused alternative to JavaScript's native array map. | [homepage](https://github.com/jonschlinkert/arr-map)
|
||||
* [arr-pluck](https://www.npmjs.com/package/arr-pluck): Retrieves the value of a specified property from all elements in the collection. | [homepage](https://github.com/jonschlinkert/arr-pluck)
|
||||
* [arr-reduce](https://www.npmjs.com/package/arr-reduce): Fast array reduce that also loops over sparse elements. | [homepage](https://github.com/jonschlinkert/arr-reduce)
|
||||
* [array-unique](https://www.npmjs.com/package/array-unique): Return an array free of duplicate values. Fastest ES5 implementation. | [homepage](https://github.com/jonschlinkert/array-unique)
|
||||
|
||||
## Contributing
|
||||
|
||||
Pull requests and stars are always welcome. For bugs and feature requests, [please create an issue](https://github.com/jonschlinkert/arr-union/issues/new).
|
||||
|
||||
## Building docs
|
||||
|
||||
Generate readme and API documentation with [verb](https://github.com/verbose/verb):
|
||||
|
||||
```sh
|
||||
$ npm i verb && npm run docs
|
||||
```
|
||||
|
||||
Or, if [verb](https://github.com/verbose/verb) is installed globally:
|
||||
|
||||
```sh
|
||||
$ verb
|
||||
```
|
||||
|
||||
## Running tests
|
||||
|
||||
Install dev dependencies:
|
||||
|
||||
```sh
|
||||
$ npm i -d && npm test
|
||||
```
|
||||
|
||||
## Author
|
||||
|
||||
**Jon Schlinkert**
|
||||
|
||||
* [github/jonschlinkert](https://github.com/jonschlinkert)
|
||||
* [twitter/jonschlinkert](http://twitter.com/jonschlinkert)
|
||||
|
||||
## License
|
||||
|
||||
Copyright © 2016 [Jon Schlinkert](https://github.com/jonschlinkert)
|
||||
Released under the [MIT license](https://github.com/jonschlinkert/arr-union/blob/master/LICENSE).
|
||||
|
||||
***
|
||||
|
||||
_This file was generated by [verb](https://github.com/verbose/verb), v0.9.0, on February 23, 2016._
|
29
node_modules/arr-union/index.js
generated
vendored
Normal file
@ -0,0 +1,29 @@
|
||||
'use strict';
|
||||
|
||||
module.exports = function union(init) {
|
||||
if (!Array.isArray(init)) {
|
||||
throw new TypeError('arr-union expects the first argument to be an array.');
|
||||
}
|
||||
|
||||
var len = arguments.length;
|
||||
var i = 0;
|
||||
|
||||
while (++i < len) {
|
||||
var arg = arguments[i];
|
||||
if (!arg) continue;
|
||||
|
||||
if (!Array.isArray(arg)) {
|
||||
arg = [arg];
|
||||
}
|
||||
|
||||
for (var j = 0; j < arg.length; j++) {
|
||||
var ele = arg[j];
|
||||
|
||||
if (init.indexOf(ele) >= 0) {
|
||||
continue;
|
||||
}
|
||||
init.push(ele);
|
||||
}
|
||||
}
|
||||
return init;
|
||||
};
|
108
node_modules/arr-union/package.json
generated
vendored
Normal file
@ -0,0 +1,108 @@
|
||||
{
|
||||
"_from": "arr-union@^3.1.0",
|
||||
"_id": "arr-union@3.1.0",
|
||||
"_inBundle": false,
|
||||
"_integrity": "sha1-45sJrqne+Gao8gbiiK9jkZuuOcQ=",
|
||||
"_location": "/arr-union",
|
||||
"_phantomChildren": {},
|
||||
"_requested": {
|
||||
"type": "range",
|
||||
"registry": true,
|
||||
"raw": "arr-union@^3.1.0",
|
||||
"name": "arr-union",
|
||||
"escapedName": "arr-union",
|
||||
"rawSpec": "^3.1.0",
|
||||
"saveSpec": null,
|
||||
"fetchSpec": "^3.1.0"
|
||||
},
|
||||
"_requiredBy": [
|
||||
"/class-utils",
|
||||
"/union-value"
|
||||
],
|
||||
"_resolved": "https://registry.npmjs.org/arr-union/-/arr-union-3.1.0.tgz",
|
||||
"_shasum": "e39b09aea9def866a8f206e288af63919bae39c4",
|
||||
"_spec": "arr-union@^3.1.0",
|
||||
"_where": "C:\\Users\\User\\Documents\\Git\\asdf-games\\node_modules\\union-value",
|
||||
"author": {
|
||||
"name": "Jon Schlinkert",
|
||||
"url": "https://github.com/jonschlinkert"
|
||||
},
|
||||
"bugs": {
|
||||
"url": "https://github.com/jonschlinkert/arr-union/issues"
|
||||
},
|
||||
"bundleDependencies": false,
|
||||
"deprecated": false,
|
||||
"description": "Combines a list of arrays, returning a single array with unique values, using strict equality for comparisons.",
|
||||
"devDependencies": {
|
||||
"ansi-bold": "^0.1.1",
|
||||
"array-union": "^1.0.1",
|
||||
"array-unique": "^0.2.1",
|
||||
"benchmarked": "^0.1.4",
|
||||
"gulp-format-md": "^0.1.7",
|
||||
"minimist": "^1.1.1",
|
||||
"mocha": "*",
|
||||
"should": "*"
|
||||
},
|
||||
"engines": {
|
||||
"node": ">=0.10.0"
|
||||
},
|
||||
"files": [
|
||||
"index.js"
|
||||
],
|
||||
"homepage": "https://github.com/jonschlinkert/arr-union",
|
||||
"keywords": [
|
||||
"add",
|
||||
"append",
|
||||
"array",
|
||||
"arrays",
|
||||
"combine",
|
||||
"concat",
|
||||
"extend",
|
||||
"union",
|
||||
"uniq",
|
||||
"unique",
|
||||
"util",
|
||||
"utility",
|
||||
"utils"
|
||||
],
|
||||
"license": "MIT",
|
||||
"main": "index.js",
|
||||
"name": "arr-union",
|
||||
"repository": {
|
||||
"type": "git",
|
||||
"url": "git+https://github.com/jonschlinkert/arr-union.git"
|
||||
},
|
||||
"scripts": {
|
||||
"test": "mocha"
|
||||
},
|
||||
"verb": {
|
||||
"run": true,
|
||||
"toc": false,
|
||||
"layout": "default",
|
||||
"tasks": [
|
||||
"readme"
|
||||
],
|
||||
"plugins": [
|
||||
"gulp-format-md"
|
||||
],
|
||||
"related": {
|
||||
"list": [
|
||||
"arr-diff",
|
||||
"arr-flatten",
|
||||
"arr-filter",
|
||||
"arr-map",
|
||||
"arr-pluck",
|
||||
"arr-reduce",
|
||||
"array-unique"
|
||||
]
|
||||
},
|
||||
"reflinks": [
|
||||
"verb",
|
||||
"array-union"
|
||||
],
|
||||
"lint": {
|
||||
"reflinks": true
|
||||
}
|
||||
},
|
||||
"version": "3.1.0"
|
||||
}
|
21
node_modules/array-unique/LICENSE
generated
vendored
Normal file
@ -0,0 +1,21 @@
|
||||
The MIT License (MIT)
|
||||
|
||||
Copyright (c) 2014-2016, Jon Schlinkert
|
||||
|
||||
Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
of this software and associated documentation files (the "Software"), to deal
|
||||
in the Software without restriction, including without limitation the rights
|
||||
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
copies of the Software, and to permit persons to whom the Software is
|
||||
furnished to do so, subject to the following conditions:
|
||||
|
||||
The above copyright notice and this permission notice shall be included in
|
||||
all copies or substantial portions of the Software.
|
||||
|
||||
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
|
||||
THE SOFTWARE.
|
77
node_modules/array-unique/README.md
generated
vendored
Normal file
@ -0,0 +1,77 @@
|
||||
# array-unique [](https://www.npmjs.com/package/array-unique) [](https://npmjs.org/package/array-unique) [](https://travis-ci.org/jonschlinkert/array-unique)
|
||||
|
||||
Remove duplicate values from an array. Fastest ES5 implementation.
|
||||
|
||||
## Install
|
||||
|
||||
Install with [npm](https://www.npmjs.com/):
|
||||
|
||||
```sh
|
||||
$ npm install --save array-unique
|
||||
```
|
||||
|
||||
## Usage
|
||||
|
||||
```js
|
||||
var unique = require('array-unique');
|
||||
|
||||
var arr = ['a', 'b', 'c', 'c'];
|
||||
console.log(unique(arr)) //=> ['a', 'b', 'c']
|
||||
console.log(arr) //=> ['a', 'b', 'c']
|
||||
|
||||
/* The above modifies the input array. To prevent that at a slight performance cost: */
|
||||
var unique = require("array-unique").immutable;
|
||||
|
||||
var arr = ['a', 'b', 'c', 'c'];
|
||||
console.log(unique(arr)) //=> ['a', 'b', 'c']
|
||||
console.log(arr) //=> ['a', 'b', 'c', 'c']
|
||||
```
|
||||
|
||||
## About
|
||||
|
||||
### Related projects
|
||||
|
||||
* [arr-diff](https://www.npmjs.com/package/arr-diff): Returns an array with only the unique values from the first array, by excluding all… [more](https://github.com/jonschlinkert/arr-diff) | [homepage](https://github.com/jonschlinkert/arr-diff "Returns an array with only the unique values from the first array, by excluding all values from additional arrays using strict equality for comparisons.")
|
||||
* [arr-flatten](https://www.npmjs.com/package/arr-flatten): Recursively flatten an array or arrays. This is the fastest implementation of array flatten. | [homepage](https://github.com/jonschlinkert/arr-flatten "Recursively flatten an array or arrays. This is the fastest implementation of array flatten.")
|
||||
* [arr-map](https://www.npmjs.com/package/arr-map): Faster, node.js focused alternative to JavaScript's native array map. | [homepage](https://github.com/jonschlinkert/arr-map "Faster, node.js focused alternative to JavaScript's native array map.")
|
||||
* [arr-pluck](https://www.npmjs.com/package/arr-pluck): Retrieves the value of a specified property from all elements in the collection. | [homepage](https://github.com/jonschlinkert/arr-pluck "Retrieves the value of a specified property from all elements in the collection.")
|
||||
* [arr-reduce](https://www.npmjs.com/package/arr-reduce): Fast array reduce that also loops over sparse elements. | [homepage](https://github.com/jonschlinkert/arr-reduce "Fast array reduce that also loops over sparse elements.")
|
||||
* [arr-union](https://www.npmjs.com/package/arr-union): Combines a list of arrays, returning a single array with unique values, using strict equality… [more](https://github.com/jonschlinkert/arr-union) | [homepage](https://github.com/jonschlinkert/arr-union "Combines a list of arrays, returning a single array with unique values, using strict equality for comparisons.")
|
||||
|
||||
### Contributing
|
||||
|
||||
Pull requests and stars are always welcome. For bugs and feature requests, [please create an issue](../../issues/new).
|
||||
|
||||
### Building docs
|
||||
|
||||
_(This document was generated by [verb-generate-readme](https://github.com/verbose/verb-generate-readme) (a [verb](https://github.com/verbose/verb) generator), please don't edit the readme directly. Any changes to the readme must be made in [.verb.md](.verb.md).)_
|
||||
|
||||
To generate the readme and API documentation with [verb](https://github.com/verbose/verb):
|
||||
|
||||
```sh
|
||||
$ npm install -g verb verb-generate-readme && verb
|
||||
```
|
||||
|
||||
### Running tests
|
||||
|
||||
Install dev dependencies:
|
||||
|
||||
```sh
|
||||
$ npm install -d && npm test
|
||||
```
|
||||
|
||||
### Author
|
||||
|
||||
**Jon Schlinkert**
|
||||
|
||||
* [github/jonschlinkert](https://github.com/jonschlinkert)
|
||||
* [twitter/jonschlinkert](http://twitter.com/jonschlinkert)
|
||||
|
||||
### License
|
||||
|
||||
Copyright © 2016, [Jon Schlinkert](https://github.com/jonschlinkert).
|
||||
Released under the [MIT license](https://github.com/jonschlinkert/array-unique/blob/master/LICENSE).
|
||||
|
||||
***
|
||||
|
||||
_This file was generated by [verb-generate-readme](https://github.com/verbose/verb-generate-readme), v0.1.28, on July 31, 2016._
|
43
node_modules/array-unique/index.js
generated
vendored
Normal file
@ -0,0 +1,43 @@
|
||||
/*!
|
||||
* array-unique <https://github.com/jonschlinkert/array-unique>
|
||||
*
|
||||
* Copyright (c) 2014-2015, Jon Schlinkert.
|
||||
* Licensed under the MIT License.
|
||||
*/
|
||||
|
||||
'use strict';
|
||||
|
||||
module.exports = function unique(arr) {
|
||||
if (!Array.isArray(arr)) {
|
||||
throw new TypeError('array-unique expects an array.');
|
||||
}
|
||||
|
||||
var len = arr.length;
|
||||
var i = -1;
|
||||
|
||||
while (i++ < len) {
|
||||
var j = i + 1;
|
||||
|
||||
for (; j < arr.length; ++j) {
|
||||
if (arr[i] === arr[j]) {
|
||||
arr.splice(j--, 1);
|
||||
}
|
||||
}
|
||||
}
|
||||
return arr;
|
||||
};
|
||||
|
||||
module.exports.immutable = function uniqueImmutable(arr) {
|
||||
if (!Array.isArray(arr)) {
|
||||
throw new TypeError('array-unique expects an array.');
|
||||
}
|
||||
|
||||
var arrLen = arr.length;
|
||||
var newArr = new Array(arrLen);
|
||||
|
||||
for (var i = 0; i < arrLen; i++) {
|
||||
newArr[i] = arr[i];
|
||||
}
|
||||
|
||||
return module.exports(newArr);
|
||||
};
|
96
node_modules/array-unique/package.json
generated
vendored
Normal file
@ -0,0 +1,96 @@
|
||||
{
|
||||
"_from": "array-unique@^0.3.2",
|
||||
"_id": "array-unique@0.3.2",
|
||||
"_inBundle": false,
|
||||
"_integrity": "sha1-qJS3XUvE9s1nnvMkSp/Y9Gri1Cg=",
|
||||
"_location": "/array-unique",
|
||||
"_phantomChildren": {},
|
||||
"_requested": {
|
||||
"type": "range",
|
||||
"registry": true,
|
||||
"raw": "array-unique@^0.3.2",
|
||||
"name": "array-unique",
|
||||
"escapedName": "array-unique",
|
||||
"rawSpec": "^0.3.2",
|
||||
"saveSpec": null,
|
||||
"fetchSpec": "^0.3.2"
|
||||
},
|
||||
"_requiredBy": [
|
||||
"/braces",
|
||||
"/extglob",
|
||||
"/micromatch",
|
||||
"/nanomatch"
|
||||
],
|
||||
"_resolved": "https://registry.npmjs.org/array-unique/-/array-unique-0.3.2.tgz",
|
||||
"_shasum": "a894b75d4bc4f6cd679ef3244a9fd8f46ae2d428",
|
||||
"_spec": "array-unique@^0.3.2",
|
||||
"_where": "C:\\Users\\User\\Documents\\Git\\asdf-games\\node_modules\\micromatch",
|
||||
"author": {
|
||||
"name": "Jon Schlinkert",
|
||||
"url": "https://github.com/jonschlinkert"
|
||||
},
|
||||
"bugs": {
|
||||
"url": "https://github.com/jonschlinkert/array-unique/issues"
|
||||
},
|
||||
"bundleDependencies": false,
|
||||
"deprecated": false,
|
||||
"description": "Remove duplicate values from an array. Fastest ES5 implementation.",
|
||||
"devDependencies": {
|
||||
"array-uniq": "^1.0.2",
|
||||
"benchmarked": "^0.1.3",
|
||||
"gulp-format-md": "^0.1.9",
|
||||
"mocha": "^2.5.3",
|
||||
"should": "^10.0.0"
|
||||
},
|
||||
"engines": {
|
||||
"node": ">=0.10.0"
|
||||
},
|
||||
"files": [
|
||||
"index.js",
|
||||
"LICENSE",
|
||||
"README.md"
|
||||
],
|
||||
"homepage": "https://github.com/jonschlinkert/array-unique",
|
||||
"keywords": [
|
||||
"array",
|
||||
"unique"
|
||||
],
|
||||
"license": "MIT",
|
||||
"main": "index.js",
|
||||
"name": "array-unique",
|
||||
"repository": {
|
||||
"type": "git",
|
||||
"url": "git+https://github.com/jonschlinkert/array-unique.git"
|
||||
},
|
||||
"scripts": {
|
||||
"test": "mocha"
|
||||
},
|
||||
"verb": {
|
||||
"toc": false,
|
||||
"layout": "default",
|
||||
"tasks": [
|
||||
"readme"
|
||||
],
|
||||
"plugins": [
|
||||
"gulp-format-md"
|
||||
],
|
||||
"related": {
|
||||
"list": [
|
||||
"arr-diff",
|
||||
"arr-union",
|
||||
"arr-flatten",
|
||||
"arr-reduce",
|
||||
"arr-map",
|
||||
"arr-pluck"
|
||||
]
|
||||
},
|
||||
"reflinks": [
|
||||
"verb",
|
||||
"verb-generate-readme"
|
||||
],
|
||||
"lint": {
|
||||
"reflinks": true
|
||||
}
|
||||
},
|
||||
"version": "0.3.2"
|
||||
}
|
21
node_modules/assign-symbols/LICENSE
generated
vendored
Normal file
@ -0,0 +1,21 @@
|
||||
The MIT License (MIT)
|
||||
|
||||
Copyright (c) 2015, Jon Schlinkert.
|
||||
|
||||
Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
of this software and associated documentation files (the "Software"), to deal
|
||||
in the Software without restriction, including without limitation the rights
|
||||
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
copies of the Software, and to permit persons to whom the Software is
|
||||
furnished to do so, subject to the following conditions:
|
||||
|
||||
The above copyright notice and this permission notice shall be included in
|
||||
all copies or substantial portions of the Software.
|
||||
|
||||
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
|
||||
THE SOFTWARE.
|
73
node_modules/assign-symbols/README.md
generated
vendored
Normal file
@ -0,0 +1,73 @@
|
||||
# assign-symbols [](http://badge.fury.io/js/assign-symbols)
|
||||
|
||||
> Assign the enumerable es6 Symbol properties from an object (or objects) to the first object passed on the arguments. Can be used as a supplement to other extend, assign or merge methods as a polyfill for the Symbols part of the es6 Object.assign method.
|
||||
|
||||
From the [Mozilla Developer docs for Symbol](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Symbol):
|
||||
|
||||
> A symbol is a unique and immutable data type and may be used as an identifier for object properties. The symbol object is an implicit object wrapper for the symbol primitive data type.
|
||||
|
||||
## Install
|
||||
|
||||
Install with [npm](https://www.npmjs.com/)
|
||||
|
||||
```sh
|
||||
$ npm i assign-symbols --save
|
||||
```
|
||||
|
||||
## Usage
|
||||
|
||||
```js
|
||||
var assignSymbols = require('assign-symbols');
|
||||
var obj = {};
|
||||
|
||||
var one = {};
|
||||
var symbolOne = Symbol('aaa');
|
||||
one[symbolOne] = 'bbb';
|
||||
|
||||
var two = {};
|
||||
var symbolTwo = Symbol('ccc');
|
||||
two[symbolTwo] = 'ddd';
|
||||
|
||||
assignSymbols(obj, one, two);
|
||||
|
||||
console.log(obj[symbolOne]);
|
||||
//=> 'bbb'
|
||||
console.log(obj[symbolTwo]);
|
||||
//=> 'ddd'
|
||||
```
|
||||
|
||||
## Similar projects
|
||||
|
||||
* [assign-deep](https://www.npmjs.com/package/assign-deep): Deeply assign the enumerable properties of source objects to a destination object. | [homepage](https://github.com/jonschlinkert/assign-deep)
|
||||
* [clone-deep](https://www.npmjs.com/package/clone-deep): Recursively (deep) clone JavaScript native types, like Object, Array, RegExp, Date as well as primitives. | [homepage](https://github.com/jonschlinkert/clone-deep)
|
||||
* [extend-shallow](https://www.npmjs.com/package/extend-shallow): Extend an object with the properties of additional objects. node.js/javascript util. | [homepage](https://github.com/jonschlinkert/extend-shallow)
|
||||
* [merge-deep](https://www.npmjs.com/package/merge-deep): Recursively merge values in a javascript object. | [homepage](https://github.com/jonschlinkert/merge-deep)
|
||||
* [mixin-deep](https://www.npmjs.com/package/mixin-deep): Deeply mix the properties of objects into the first object. Like merge-deep, but doesn't clone. | [homepage](https://github.com/jonschlinkert/mixin-deep)
|
||||
|
||||
## Running tests
|
||||
|
||||
Install dev dependencies:
|
||||
|
||||
```sh
|
||||
$ npm i -d && npm test
|
||||
```
|
||||
|
||||
## Contributing
|
||||
|
||||
Pull requests and stars are always welcome. For bugs and feature requests, [please create an issue](https://github.com/jonschlinkert/assign-symbols/issues/new).
|
||||
|
||||
## Author
|
||||
|
||||
**Jon Schlinkert**
|
||||
|
||||
+ [github/jonschlinkert](https://github.com/jonschlinkert)
|
||||
+ [twitter/jonschlinkert](http://twitter.com/jonschlinkert)
|
||||
|
||||
## License
|
||||
|
||||
Copyright © 2015 Jon Schlinkert
|
||||
Released under the MIT license.
|
||||
|
||||
***
|
||||
|
||||
_This file was generated by [verb-cli](https://github.com/assemble/verb-cli) on November 06, 2015._
|
40
node_modules/assign-symbols/index.js
generated
vendored
Normal file
@ -0,0 +1,40 @@
|
||||
/*!
|
||||
* assign-symbols <https://github.com/jonschlinkert/assign-symbols>
|
||||
*
|
||||
* Copyright (c) 2015, Jon Schlinkert.
|
||||
* Licensed under the MIT License.
|
||||
*/
|
||||
|
||||
'use strict';
|
||||
|
||||
module.exports = function(receiver, objects) {
|
||||
if (receiver === null || typeof receiver === 'undefined') {
|
||||
throw new TypeError('expected first argument to be an object.');
|
||||
}
|
||||
|
||||
if (typeof objects === 'undefined' || typeof Symbol === 'undefined') {
|
||||
return receiver;
|
||||
}
|
||||
|
||||
if (typeof Object.getOwnPropertySymbols !== 'function') {
|
||||
return receiver;
|
||||
}
|
||||
|
||||
var isEnumerable = Object.prototype.propertyIsEnumerable;
|
||||
var target = Object(receiver);
|
||||
var len = arguments.length, i = 0;
|
||||
|
||||
while (++i < len) {
|
||||
var provider = Object(arguments[i]);
|
||||
var names = Object.getOwnPropertySymbols(provider);
|
||||
|
||||
for (var j = 0; j < names.length; j++) {
|
||||
var key = names[j];
|
||||
|
||||
if (isEnumerable.call(provider, key)) {
|
||||
target[key] = provider[key];
|
||||
}
|
||||
}
|
||||
}
|
||||
return target;
|
||||
};
|
71
node_modules/assign-symbols/package.json
generated
vendored
Normal file
@ -0,0 +1,71 @@
|
||||
{
|
||||
"_from": "assign-symbols@^1.0.0",
|
||||
"_id": "assign-symbols@1.0.0",
|
||||
"_inBundle": false,
|
||||
"_integrity": "sha1-WWZ/QfrdTyDMvCu5a41Pf3jsA2c=",
|
||||
"_location": "/assign-symbols",
|
||||
"_phantomChildren": {},
|
||||
"_requested": {
|
||||
"type": "range",
|
||||
"registry": true,
|
||||
"raw": "assign-symbols@^1.0.0",
|
||||
"name": "assign-symbols",
|
||||
"escapedName": "assign-symbols",
|
||||
"rawSpec": "^1.0.0",
|
||||
"saveSpec": null,
|
||||
"fetchSpec": "^1.0.0"
|
||||
},
|
||||
"_requiredBy": [
|
||||
"/extend-shallow"
|
||||
],
|
||||
"_resolved": "https://registry.npmjs.org/assign-symbols/-/assign-symbols-1.0.0.tgz",
|
||||
"_shasum": "59667f41fadd4f20ccbc2bb96b8d4f7f78ec0367",
|
||||
"_spec": "assign-symbols@^1.0.0",
|
||||
"_where": "C:\\Users\\User\\Documents\\Git\\asdf-games\\node_modules\\extend-shallow",
|
||||
"author": {
|
||||
"name": "Jon Schlinkert",
|
||||
"url": "https://github.com/jonschlinkert"
|
||||
},
|
||||
"bugs": {
|
||||
"url": "https://github.com/jonschlinkert/assign-symbols/issues"
|
||||
},
|
||||
"bundleDependencies": false,
|
||||
"deprecated": false,
|
||||
"description": "Assign the enumerable es6 Symbol properties from an object (or objects) to the first object passed on the arguments. Can be used as a supplement to other extend, assign or merge methods as a polyfill for the Symbols part of the es6 Object.assign method.",
|
||||
"devDependencies": {
|
||||
"mocha": "^3.0.0"
|
||||
},
|
||||
"engines": {
|
||||
"node": ">=0.10.0"
|
||||
},
|
||||
"files": [
|
||||
"index.js"
|
||||
],
|
||||
"homepage": "https://github.com/jonschlinkert/assign-symbols",
|
||||
"keywords": [
|
||||
"assign",
|
||||
"symbols"
|
||||
],
|
||||
"license": "MIT",
|
||||
"main": "index.js",
|
||||
"name": "assign-symbols",
|
||||
"repository": {
|
||||
"type": "git",
|
||||
"url": "git+https://github.com/jonschlinkert/assign-symbols.git"
|
||||
},
|
||||
"scripts": {
|
||||
"test": "mocha"
|
||||
},
|
||||
"verb": {
|
||||
"related": {
|
||||
"list": [
|
||||
"assign-deep",
|
||||
"mixin-deep",
|
||||
"merge-deep",
|
||||
"extend-shallow",
|
||||
"clone-deep"
|
||||
]
|
||||
}
|
||||
},
|
||||
"version": "1.0.0"
|
||||
}
|
52
node_modules/async-each/README.md
generated
vendored
Normal file
@ -0,0 +1,52 @@
|
||||
# async-each
|
||||
|
||||
No-bullshit, ultra-simple, 35-lines-of-code async parallel forEach function for JavaScript.
|
||||
|
||||
We don't need junky 30K async libs. Really.
|
||||
|
||||
For browsers and node.js.
|
||||
|
||||
## Installation
|
||||
* Just include async-each before your scripts.
|
||||
* `npm install async-each` if you’re using node.js.
|
||||
|
||||
## Usage
|
||||
|
||||
* `each(array, iterator, callback);` — `Array`, `Function`, `(optional) Function`
|
||||
* `iterator(item, next)` receives current item and a callback that will mark the item as done. `next` callback receives optional `error, transformedItem` arguments.
|
||||
* `callback(error, transformedArray)` optionally receives first error and transformed result `Array`.
|
||||
|
||||
```javascript
|
||||
var each = require('async-each');
|
||||
each(['a.js', 'b.js', 'c.js'], fs.readFile, function(error, contents) {
|
||||
if (error) console.error(error);
|
||||
console.log('Contents for a, b and c:', contents);
|
||||
});
|
||||
|
||||
// Alternatively in browser:
|
||||
asyncEach(list, fn, callback);
|
||||
```
|
||||
|
||||
## License
|
||||
|
||||
The MIT License (MIT)
|
||||
|
||||
Copyright (c) 2016 Paul Miller [(paulmillr.com)](http://paulmillr.com)
|
||||
|
||||
Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
of this software and associated documentation files (the “Software”), to deal
|
||||
in the Software without restriction, including without limitation the rights
|
||||
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
copies of the Software, and to permit persons to whom the Software is
|
||||
furnished to do so, subject to the following conditions:
|
||||
|
||||
The above copyright notice and this permission notice shall be included in
|
||||
all copies or substantial portions of the Software.
|
||||
|
||||
THE SOFTWARE IS PROVIDED “AS IS”, WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
|
||||
THE SOFTWARE.
|
38
node_modules/async-each/index.js
generated
vendored
Normal file
@ -0,0 +1,38 @@
|
||||
// async-each MIT license (by Paul Miller from https://paulmillr.com).
|
||||
(function(globals) {
|
||||
'use strict';
|
||||
var each = function(items, next, callback) {
|
||||
if (!Array.isArray(items)) throw new TypeError('each() expects array as first argument');
|
||||
if (typeof next !== 'function') throw new TypeError('each() expects function as second argument');
|
||||
if (typeof callback !== 'function') callback = Function.prototype; // no-op
|
||||
|
||||
if (items.length === 0) return callback(undefined, items);
|
||||
|
||||
var transformed = new Array(items.length);
|
||||
var count = 0;
|
||||
var returned = false;
|
||||
|
||||
items.forEach(function(item, index) {
|
||||
next(item, function(error, transformedItem) {
|
||||
if (returned) return;
|
||||
if (error) {
|
||||
returned = true;
|
||||
return callback(error);
|
||||
}
|
||||
transformed[index] = transformedItem;
|
||||
count += 1;
|
||||
if (count === items.length) return callback(undefined, transformed);
|
||||
});
|
||||
});
|
||||
};
|
||||
|
||||
if (typeof define !== 'undefined' && define.amd) {
|
||||
define([], function() {
|
||||
return each;
|
||||
}); // RequireJS
|
||||
} else if (typeof module !== 'undefined' && module.exports) {
|
||||
module.exports = each; // CommonJS
|
||||
} else {
|
||||
globals.asyncEach = each; // <script>
|
||||
}
|
||||
})(this);
|
63
node_modules/async-each/package.json
generated
vendored
Normal file
@ -0,0 +1,63 @@
|
||||
{
|
||||
"_from": "async-each@^1.0.1",
|
||||
"_id": "async-each@1.0.3",
|
||||
"_inBundle": false,
|
||||
"_integrity": "sha512-z/WhQ5FPySLdvREByI2vZiTWwCnF0moMJ1hK9YQwDTHKh6I7/uSckMetoRGb5UBZPC1z0jlw+n/XCgjeH7y1AQ==",
|
||||
"_location": "/async-each",
|
||||
"_phantomChildren": {},
|
||||
"_requested": {
|
||||
"type": "range",
|
||||
"registry": true,
|
||||
"raw": "async-each@^1.0.1",
|
||||
"name": "async-each",
|
||||
"escapedName": "async-each",
|
||||
"rawSpec": "^1.0.1",
|
||||
"saveSpec": null,
|
||||
"fetchSpec": "^1.0.1"
|
||||
},
|
||||
"_requiredBy": [
|
||||
"/chokidar"
|
||||
],
|
||||
"_resolved": "https://registry.npmjs.org/async-each/-/async-each-1.0.3.tgz",
|
||||
"_shasum": "b727dbf87d7651602f06f4d4ac387f47d91b0cbf",
|
||||
"_spec": "async-each@^1.0.1",
|
||||
"_where": "C:\\Users\\User\\Documents\\Git\\asdf-games\\node_modules\\chokidar",
|
||||
"author": {
|
||||
"name": "Paul Miller",
|
||||
"url": "https://paulmillr.com/"
|
||||
},
|
||||
"bugs": {
|
||||
"url": "https://github.com/paulmillr/async-each/issues"
|
||||
},
|
||||
"bundleDependencies": false,
|
||||
"dependencies": {},
|
||||
"deprecated": false,
|
||||
"description": "No-bullshit, ultra-simple, 35-lines-of-code async parallel forEach / map function for JavaScript.",
|
||||
"files": [
|
||||
"index.js"
|
||||
],
|
||||
"homepage": "https://github.com/paulmillr/async-each/",
|
||||
"keywords": [
|
||||
"async",
|
||||
"forEach",
|
||||
"each",
|
||||
"map",
|
||||
"asynchronous",
|
||||
"iteration",
|
||||
"iterate",
|
||||
"loop",
|
||||
"parallel",
|
||||
"concurrent",
|
||||
"array",
|
||||
"flow",
|
||||
"control flow"
|
||||
],
|
||||
"license": "MIT",
|
||||
"main": "index.js",
|
||||
"name": "async-each",
|
||||
"repository": {
|
||||
"type": "git",
|
||||
"url": "git://github.com/paulmillr/async-each.git"
|
||||
},
|
||||
"version": "1.0.3"
|
||||
}
|
2
node_modules/async-limiter/.eslintignore
generated
vendored
Normal file
@ -0,0 +1,2 @@
|
||||
coverage
|
||||
.nyc_output
|
10
node_modules/async-limiter/.nycrc
generated
vendored
Normal file
@ -0,0 +1,10 @@
|
||||
{
|
||||
"check-coverage": false,
|
||||
"lines": 99,
|
||||
"statements": 99,
|
||||
"functions": 99,
|
||||
"branches": 99,
|
||||
"include": [
|
||||
"index.js"
|
||||
]
|
||||
}
|
9
node_modules/async-limiter/.travis.yml
generated
vendored
Normal file
@ -0,0 +1,9 @@
|
||||
language: node_js
|
||||
node_js:
|
||||
- "6"
|
||||
- "8"
|
||||
- "10"
|
||||
- "node"
|
||||
script: npm run travis
|
||||
cache:
|
||||
yarn: true
|
8
node_modules/async-limiter/LICENSE
generated
vendored
Normal file
@ -0,0 +1,8 @@
|
||||
The MIT License (MIT)
|
||||
Copyright (c) 2017 Samuel Reed <samuel.trace.reed@gmail.com>
|
||||
|
||||
Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions:
|
||||
|
||||
The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software.
|
||||
|
||||
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
67
node_modules/async-limiter/index.js
generated
vendored
Normal file
@ -0,0 +1,67 @@
|
||||
'use strict';
|
||||
|
||||
function Queue(options) {
|
||||
if (!(this instanceof Queue)) {
|
||||
return new Queue(options);
|
||||
}
|
||||
|
||||
options = options || {};
|
||||
this.concurrency = options.concurrency || Infinity;
|
||||
this.pending = 0;
|
||||
this.jobs = [];
|
||||
this.cbs = [];
|
||||
this._done = done.bind(this);
|
||||
}
|
||||
|
||||
var arrayAddMethods = [
|
||||
'push',
|
||||
'unshift',
|
||||
'splice'
|
||||
];
|
||||
|
||||
arrayAddMethods.forEach(function(method) {
|
||||
Queue.prototype[method] = function() {
|
||||
var methodResult = Array.prototype[method].apply(this.jobs, arguments);
|
||||
this._run();
|
||||
return methodResult;
|
||||
};
|
||||
});
|
||||
|
||||
Object.defineProperty(Queue.prototype, 'length', {
|
||||
get: function() {
|
||||
return this.pending + this.jobs.length;
|
||||
}
|
||||
});
|
||||
|
||||
Queue.prototype._run = function() {
|
||||
if (this.pending === this.concurrency) {
|
||||
return;
|
||||
}
|
||||
if (this.jobs.length) {
|
||||
var job = this.jobs.shift();
|
||||
this.pending++;
|
||||
job(this._done);
|
||||
this._run();
|
||||
}
|
||||
|
||||
if (this.pending === 0) {
|
||||
while (this.cbs.length !== 0) {
|
||||
var cb = this.cbs.pop();
|
||||
process.nextTick(cb);
|
||||
}
|
||||
}
|
||||
};
|
||||
|
||||
Queue.prototype.onDone = function(cb) {
|
||||
if (typeof cb === 'function') {
|
||||
this.cbs.push(cb);
|
||||
this._run();
|
||||
}
|
||||
};
|
||||
|
||||
function done() {
|
||||
this.pending--;
|
||||
this._run();
|
||||
}
|
||||
|
||||
module.exports = Queue;
|